Files Test
By Mickey Arnold
starstarstarstarstarstarstarstarstarstar
Last updated 10 months ago
20 Questions
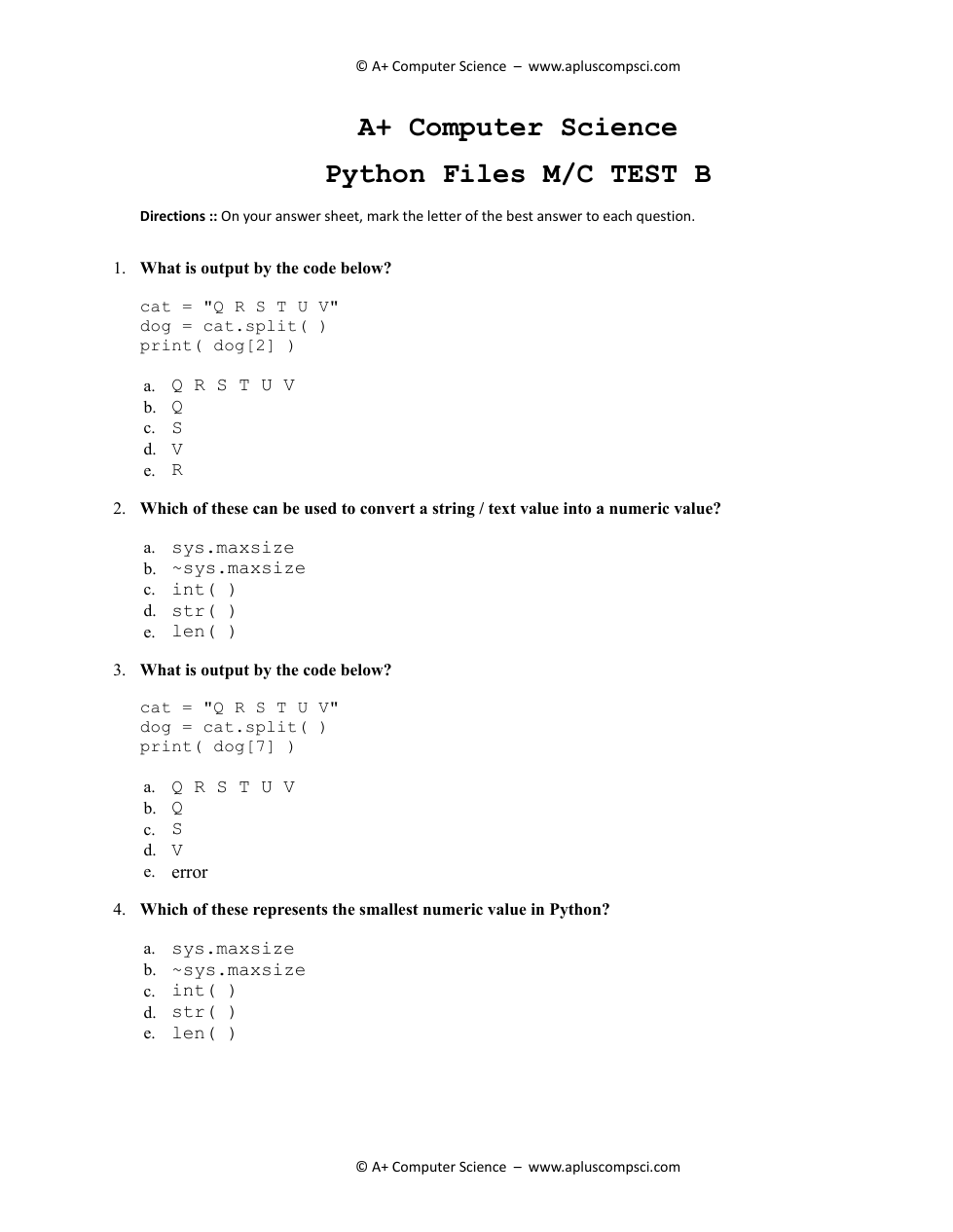
1 point
1
Question 1
1.
What is output by the code below?
cat = "Q R S T U V"
dog = cat.split()
print(dog[2])
What is output by the code below?
cat = "Q R S T U V"
dog = cat.split()
print(dog[2])
1 point
1
Question 2
2.
Which of these can be used to convert a string / text value into a numeric value?
Which of these can be used to convert a string / text value into a numeric value?
1 point
1
Question 3
3.
What is output by the code below?
cat = "Q R S T U V"
dog = cat.split()
print(dog[7])
What is output by the code below?
cat = "Q R S T U V"
dog = cat.split()
print(dog[7])
1 point
1
Question 4
4.
Which of these represents the smallest numeric value in Python?
Which of these represents the smallest numeric value in Python?
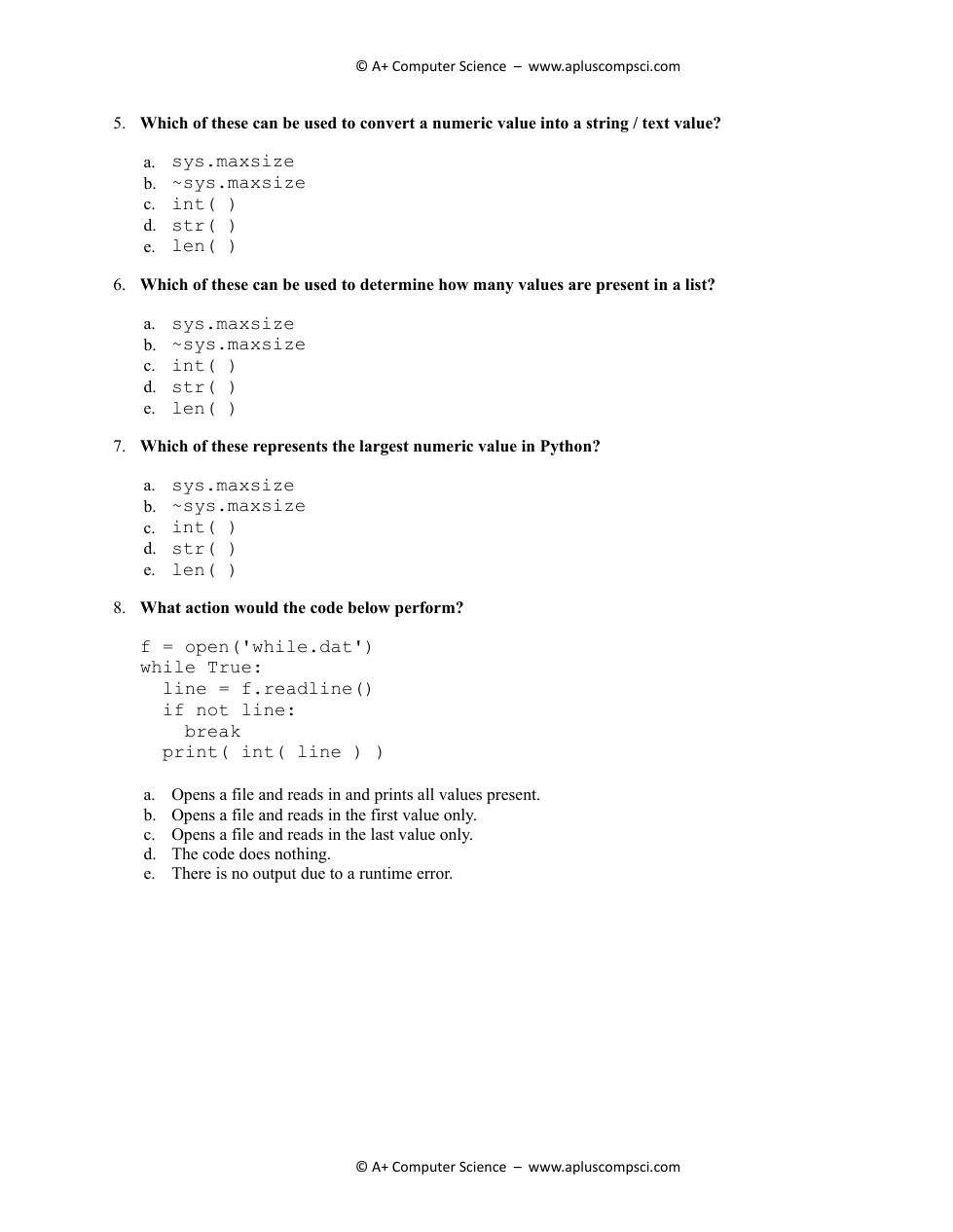
1 point
1
Question 5
5.
Question 5: Which of these can be used to convert a numeric value into a string / text value?
Question 5: Which of these can be used to convert a numeric value into a string / text value?
1 point
1
Question 6
6.
Question 6: Which of these can be used to determine how many values are present in a list?
Question 6: Which of these can be used to determine how many values are present in a list?
1 point
1
Question 7
7.
Question 7: Which of these represents the largest numeric value in Python?
Question 7: Which of these represents the largest numeric value in Python?
1 point
1
Question 8
8.
Question 8: What action would the code below perform?
f = open('while.dat')
while True:
line = f.readline()
if not line:
break
print( int( line ) )
Question 8: What action would the code below perform?
f = open('while.dat')
while True:
line = f.readline()
if not line:
break
print( int( line ) )
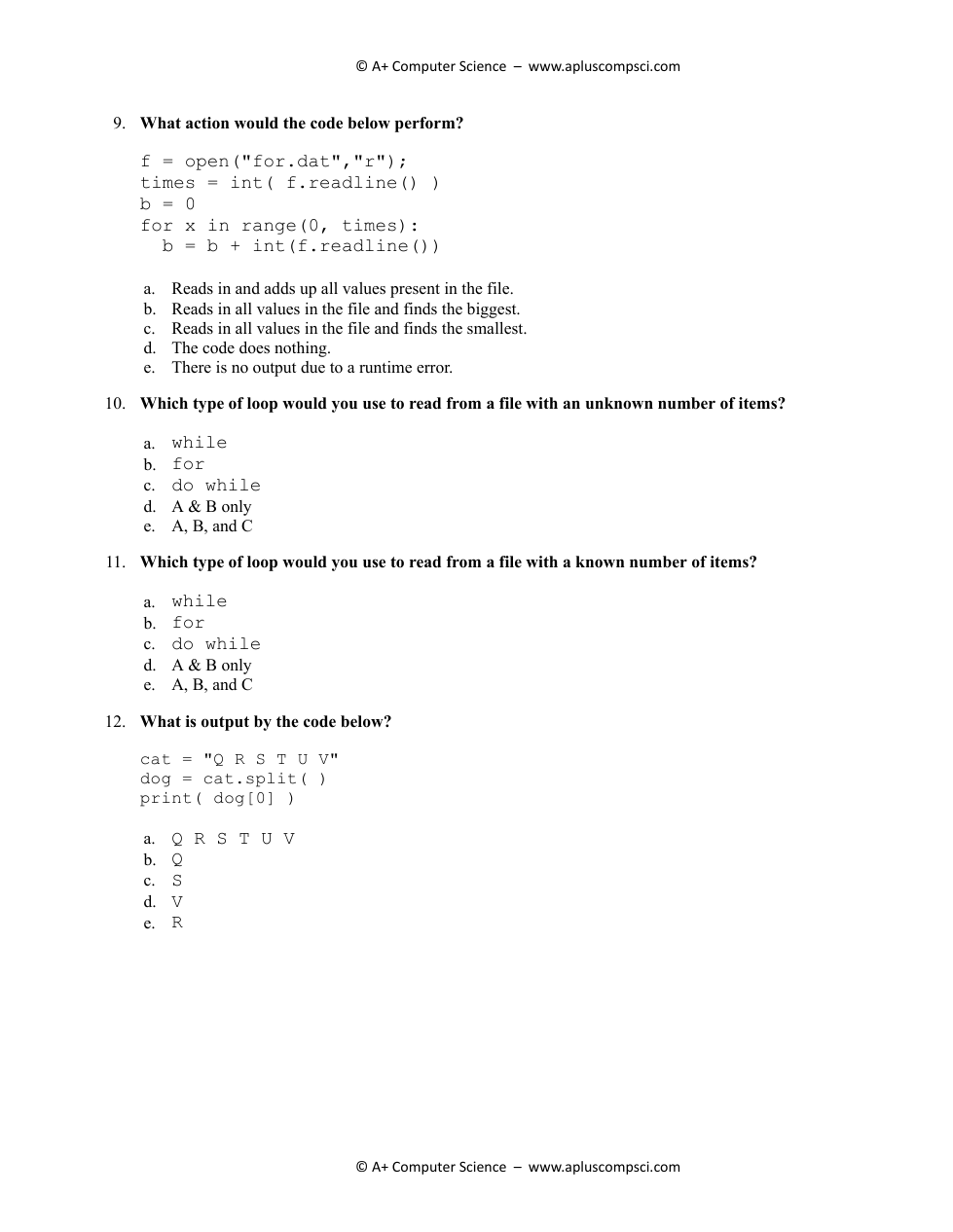
1 point
1
Question 9
9.
What action would the code below perform?
f = open("for.dat","r");
times = int( f.readline() )
b = 0
for x in range(0, times):
b = b + int(f.readline())
What action would the code below perform?
f = open("for.dat","r");
times = int( f.readline() )
b = 0
for x in range(0, times):
b = b + int(f.readline())
1 point
1
Question 10
10.
Which type of loop would you use to read from a file with an unknown number of items?
Which type of loop would you use to read from a file with an unknown number of items?
1 point
1
Question 11
11.
Which type of loop would you use to read from a file with a known number of items?
Which type of loop would you use to read from a file with a known number of items?
1 point
1
Question 12
12.
What is output by the code below?
cat = "Q R S T U V"
dog = cat.split( )
print( dog[0] )
What is output by the code below?
cat = "Q R S T U V"
dog = cat.split( )
print( dog[0] )
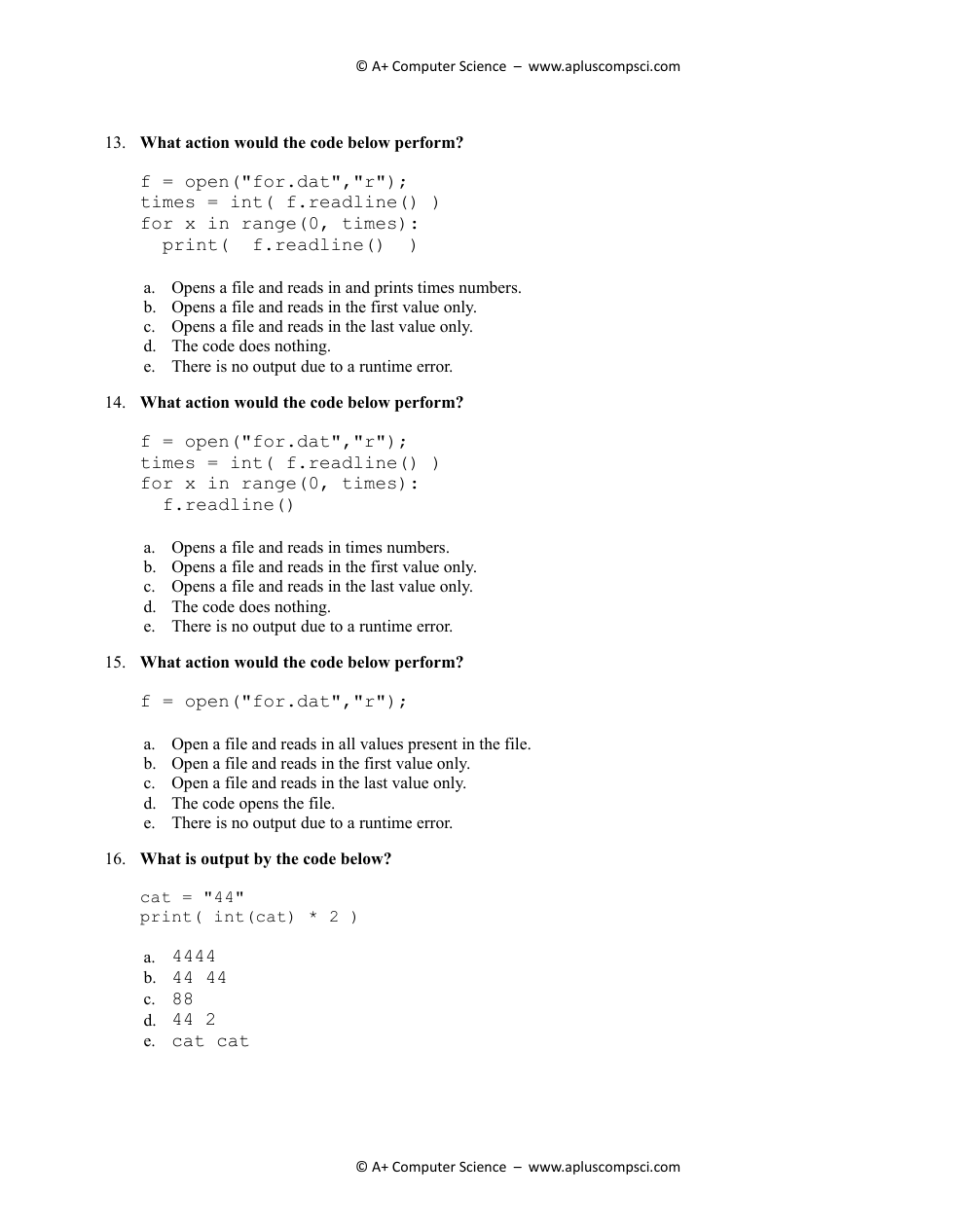
1 point
1
Question 13
13.
What action would the code below perform?f = open("for.dat","r");times = int( f.readline() )for x in range(0, times): print( f.readline() )
What action would the code below perform?
f = open("for.dat","r");
times = int( f.readline() )
for x in range(0, times):
print( f.readline() )
1 point
1
Question 14
14.
What action would the code below perform?f = open("for.dat","r");times = int( f.readline() )for x in range(0, times): f.readline()
What action would the code below perform?
f = open("for.dat","r");
times = int( f.readline() )
for x in range(0, times):
f.readline()
1 point
1
Question 15
15.
What action would the code below perform?
f = open("for.dat","r");
What action would the code below perform?
f = open("for.dat","r");
1 point
1
Question 16
16.
What is output by the code below?
cat = "44"print( int(cat) * 2 )
What is output by the code below?
cat = "44"
print( int(cat) * 2 )
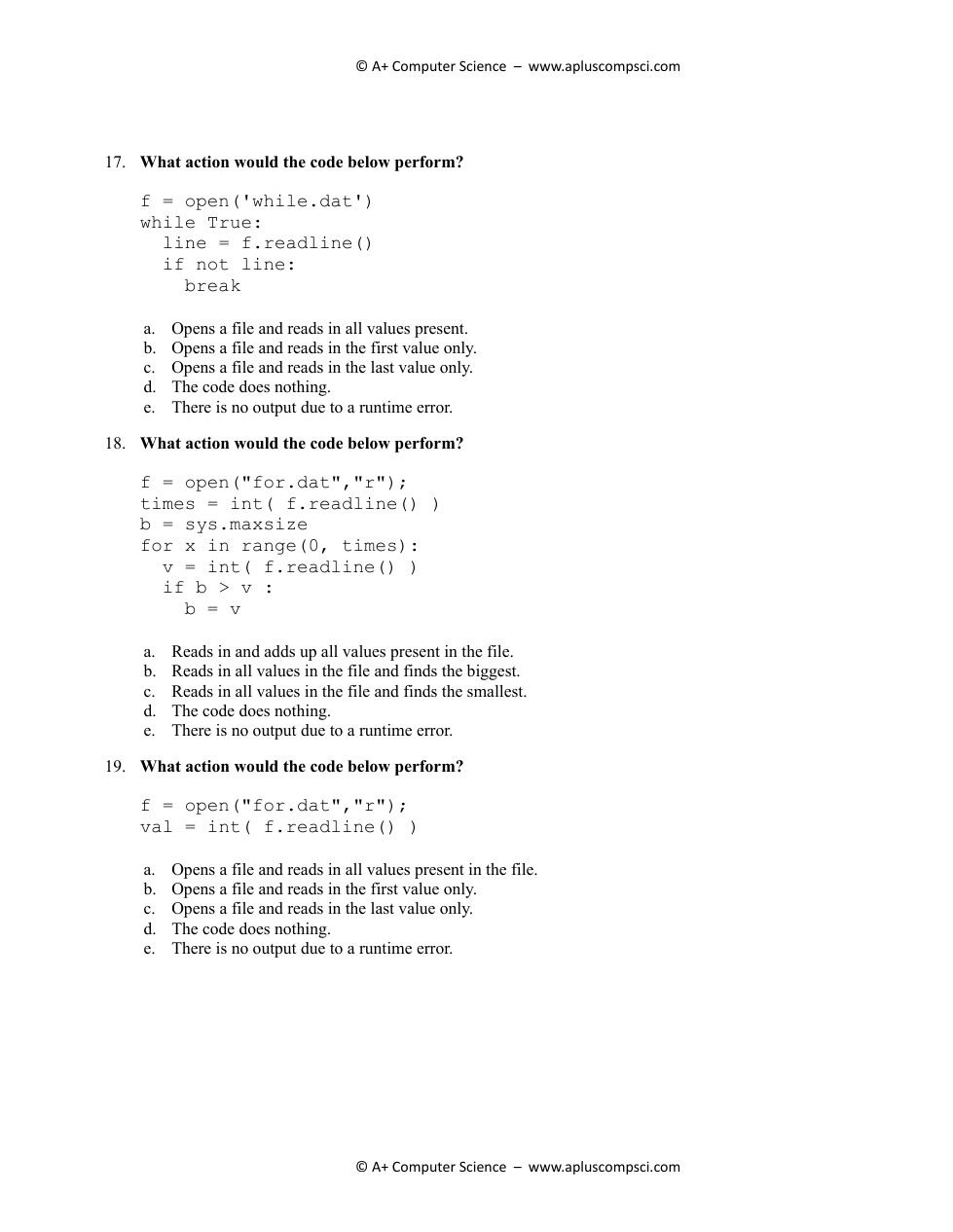
1 point
1
Question 17
17.
What action would the code below perform?
f = open('while.dat')
while True:
line = f.readline()
if not line:
break
What action would the code below perform?
f = open('while.dat')
while True:
line = f.readline()
if not line:
break
1 point
1
Question 18
18.
What action would the code below perform?
f = open("for.dat","r");
times = int( f.readline() )
b = sys.maxsize
for x in range(0, times):
v = int( f.readline() )
if b > v :
b = v
What action would the code below perform?
f = open("for.dat","r");
times = int( f.readline() )
b = sys.maxsize
for x in range(0, times):
v = int( f.readline() )
if b > v :
b = v
1 point
1
Question 19
19.
What action would the code below perform?
f = open("for.dat","r");
val = int( f.readline() )
What action would the code below perform?
f = open("for.dat","r");
val = int( f.readline() )
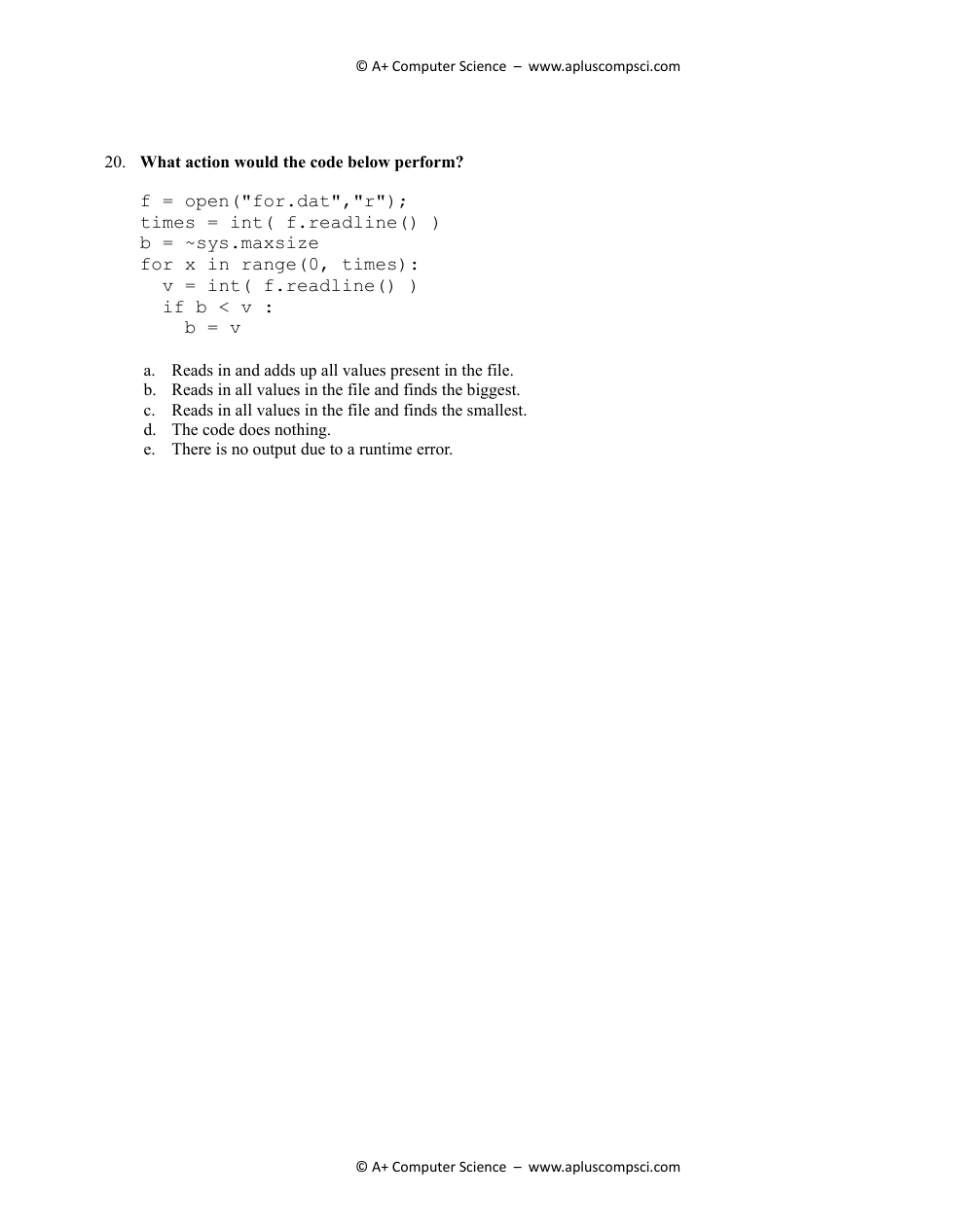
1 point
1
Question 20
20.
Question 20: What action would the code below perform?
python
f = open("for.dat","r");
times = int(f.readline())
b = ~sys.maxsize
for x in range(0, times):
v = int(f.readline())
if b < v :
b = v
Question 20: What action would the code below perform?
python
f = open("for.dat","r");
times = int(f.readline())
b = ~sys.maxsize
for x in range(0, times):
v = int(f.readline())
if b < v :
b = v