Arrays Test Part 1
By Mickey Arnold
starstarstarstarstarstarstarstarstarstar
Last updated 10 months ago
40 Questions
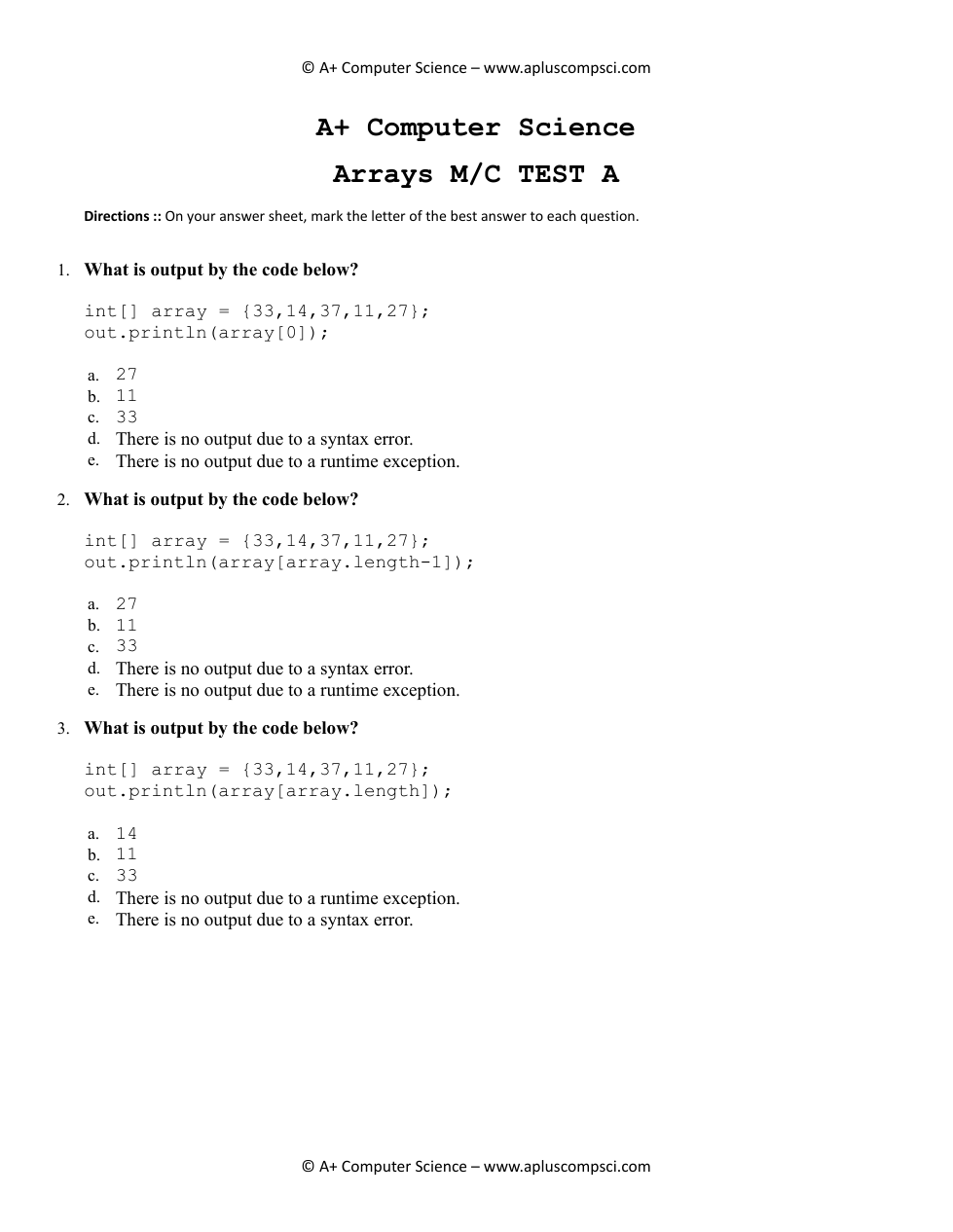
1 point
1
Question 1
1.
What is output by the code below? int[] array = {33,14,37,11,27}; out.println(array[0]);
What is output by the code below? int[] array = {33,14,37,11,27}; out.println(array[0]);
1 point
1
Question 2
2.
What is output by the code below? int[] array = {33,14,37,11,27}; out.println(array[array.length-1]);
What is output by the code below? int[] array = {33,14,37,11,27}; out.println(array[array.length-1]);
1 point
1
Question 3
3.
What is output by the code below? int[] array = {33,14,37,11,27}; out.println(array[array.length]);
What is output by the code below? int[] array = {33,14,37,11,27}; out.println(array[array.length]);
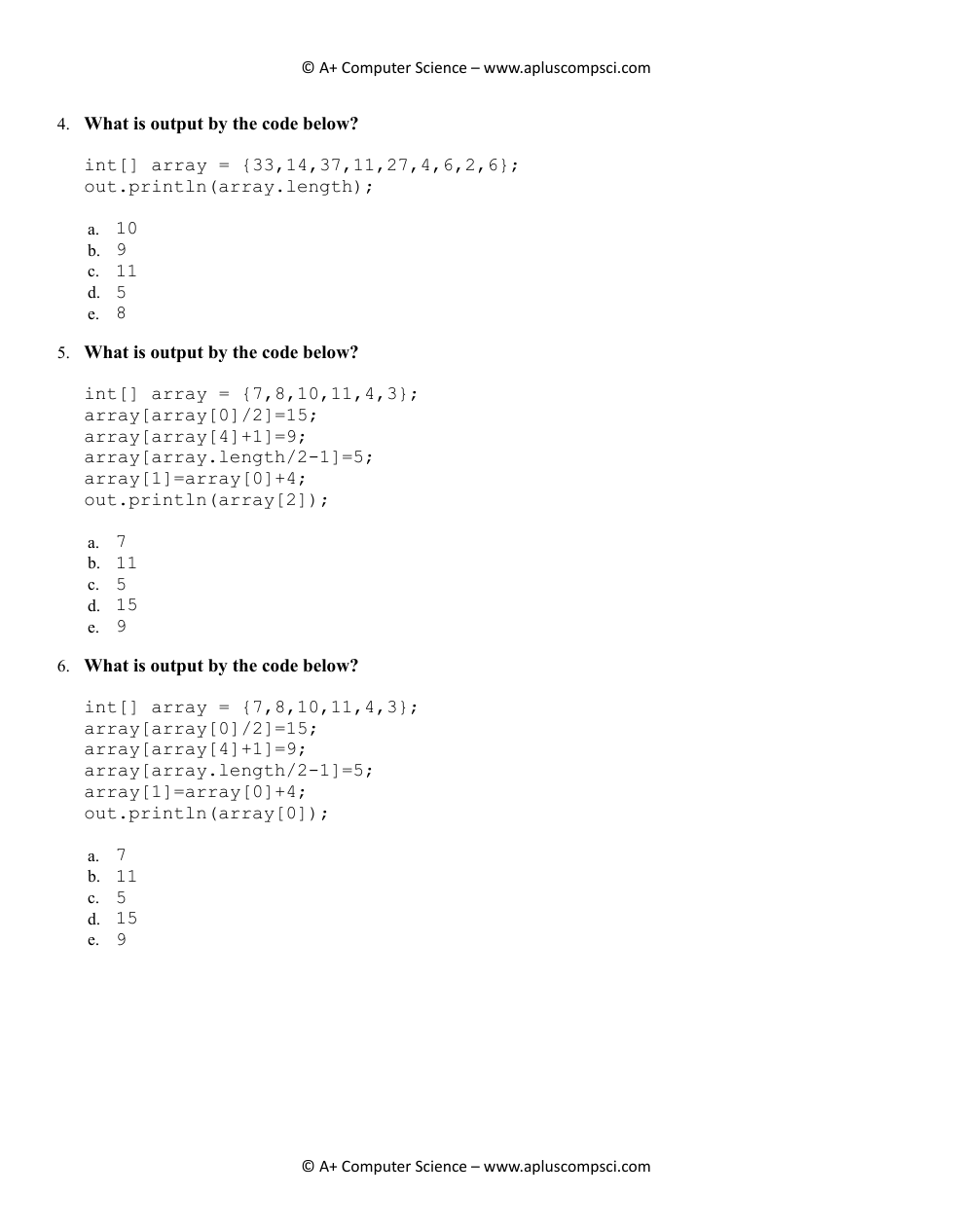
1 point
1
Question 4
4.
What is output by the code below?
int[] array = {33,14,37,11,27,4,6,2,6};
out.println(array.length);
What is output by the code below?
int[] array = {33,14,37,11,27,4,6,2,6};
out.println(array.length);
1 point
1
Question 5
5.
What is output by the code below?
int[] array = {7,8,10,11,4,3};
array[array[0]/2]=15;
array[array[4]+1]=9;
array[array.length/2-1]=5;
array[1]=array[0]+4;
out.println(array[2]);
What is output by the code below?
int[] array = {7,8,10,11,4,3};
array[array[0]/2]=15;
array[array[4]+1]=9;
array[array.length/2-1]=5;
array[1]=array[0]+4;
out.println(array[2]);
1 point
1
Question 6
6.
What is output by the code below?
int[] array = {7,8,10,11,4,3};
array[array[0]/2]=15;
array[array[4]+1]=9;
array[array.length/2-1]=5;
array[1]=array[0]+4;
out.println(array[0]);
What is output by the code below?
int[] array = {7,8,10,11,4,3};
array[array[0]/2]=15;
array[array[4]+1]=9;
array[array.length/2-1]=5;
array[1]=array[0]+4;
out.println(array[0]);
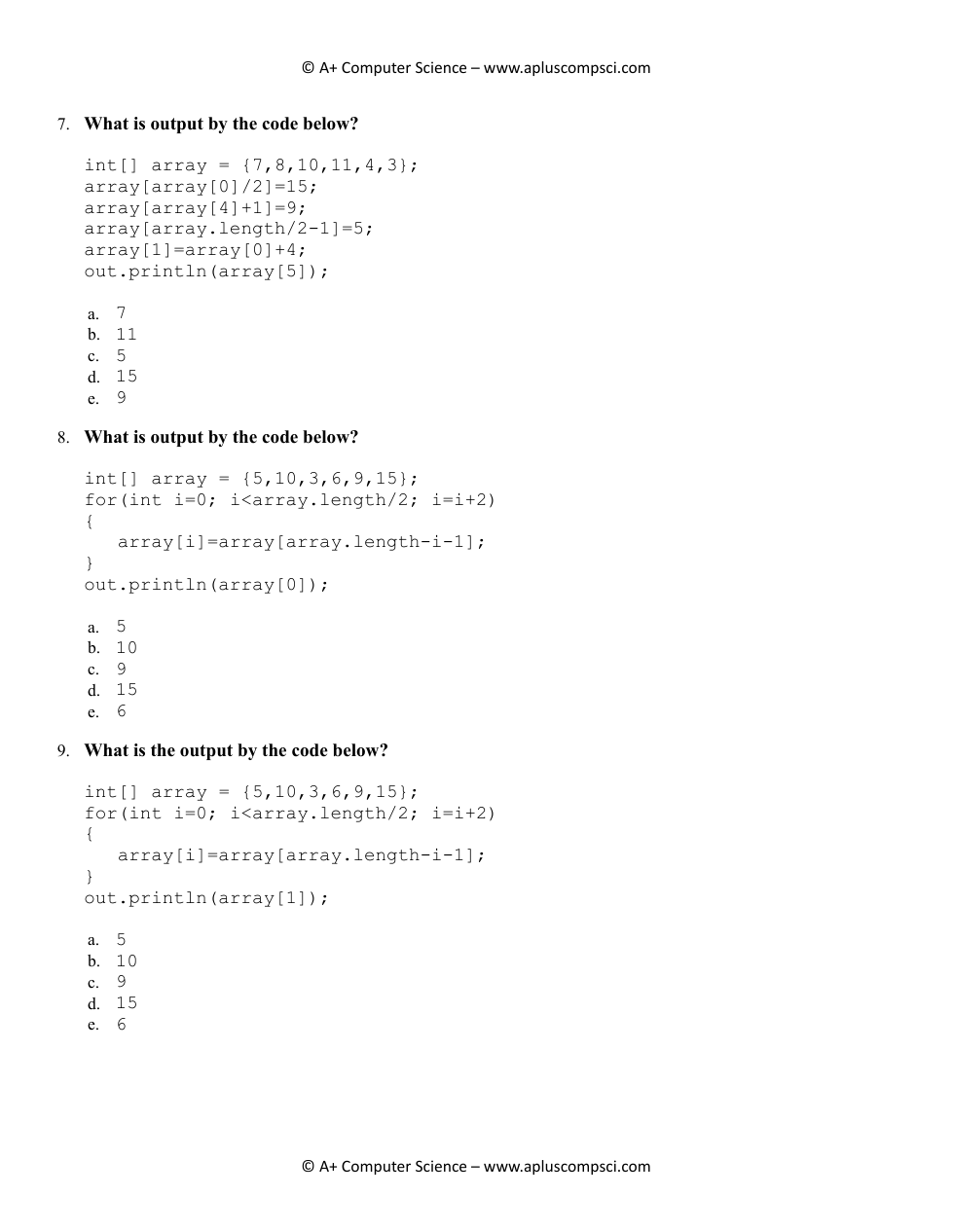
1 point
1
Question 7
7.
What is output by the code below? int[] array = {7,8,10,11,4,3}; array[array[0]/2]=15; array[array[4]+1]=9; array[array.length/2-1]=5; array[1]=array[0]+4; out.println(array[5]);
What is output by the code below? int[] array = {7,8,10,11,4,3}; array[array[0]/2]=15; array[array[4]+1]=9; array[array.length/2-1]=5; array[1]=array[0]+4; out.println(array[5]);
1 point
1
Question 8
8.
What is output by the code below?
int[] array = {5,10,3,6,9,15}; for(int i=0; i<array.length/2; i=i+2){ array[i]=array[array.length-i-1]; }
out.println(array[0]);
What is output by the code below?
int[] array = {5,10,3,6,9,15};
for(int i=0; i<array.length/2; i=i+2)
{
array[i]=array[array.length-i-1];
}
out.println(array[0]);
1 point
1
Question 9
9.
What is the output by the code below? int[] array = {5,10,3,6,9,15}; for(int i=0; i<array.length/2; i=i+2) { array[i]=array[array.length-i-1]; }
out.println(array[1]);
What is the output by the code below? int[] array = {5,10,3,6,9,15};
for(int i=0; i<array.length/2; i=i+2)
{
array[i]=array[array.length-i-1];
}
out.println(array[1]);
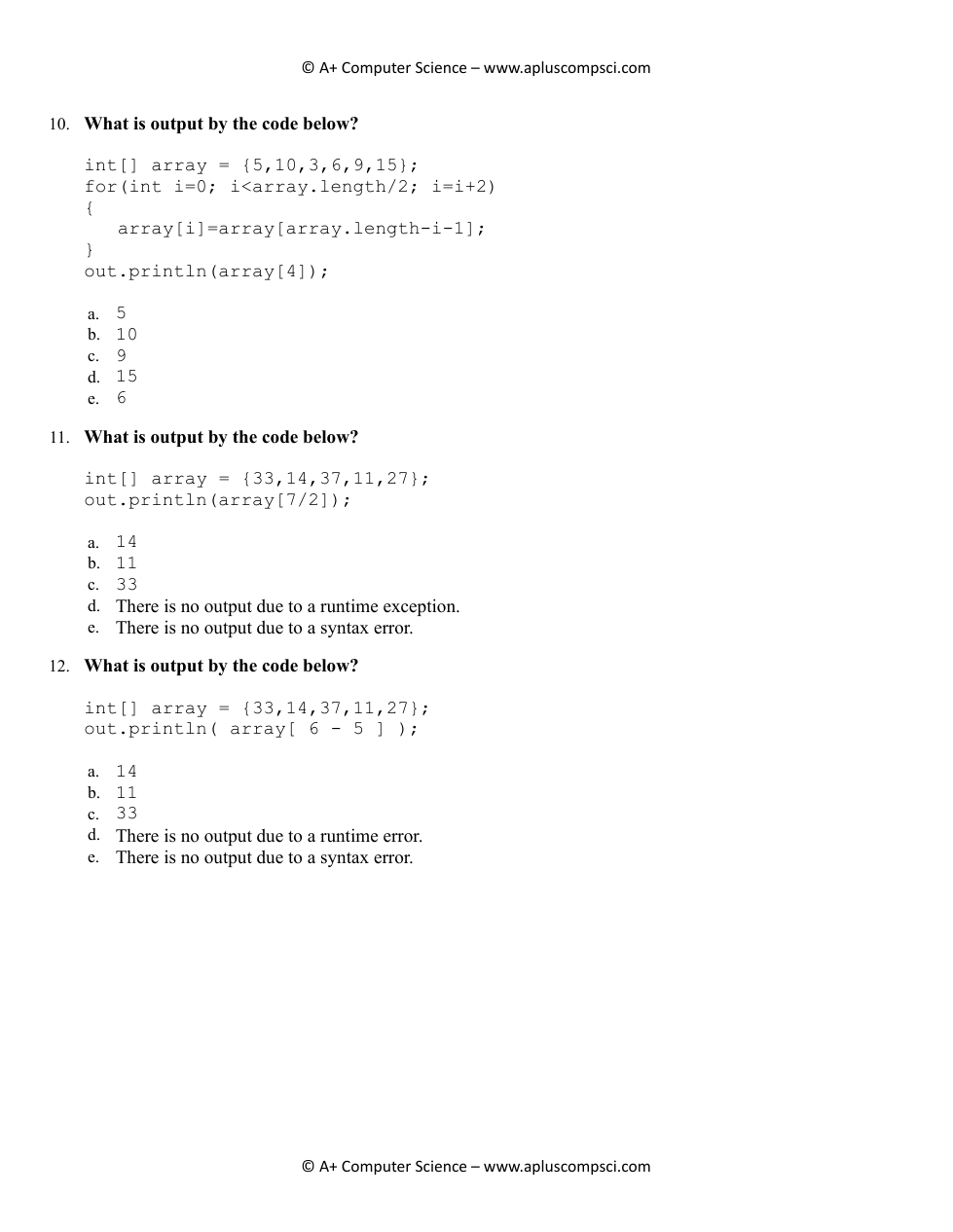
1 point
1
Question 10
10.
What is output by the code below?
int[] array = {5,10,3,6,9,15};
for(int i=0; i<array.length/2; i=i+2)
{
array[i]=array[array.length-i-1];
}
out.println(array[4]);
What is output by the code below?
int[] array = {5,10,3,6,9,15};
for(int i=0; i<array.length/2; i=i+2)
{
array[i]=array[array.length-i-1];
}
out.println(array[4]);
1 point
1
Question 11
11.
What is output by the code below?
int[] array = {33,14,37,11,27};
out.println(array[7/2]);
What is output by the code below?
int[] array = {33,14,37,11,27};
out.println(array[7/2]);
1 point
1
Question 12
12.
What is output by the code below?
int[] array = {33,14,37,11,27};
out.println( array[ 6 - 5 ] );
What is output by the code below?
int[] array = {33,14,37,11,27};
out.println( array[ 6 - 5 ] );
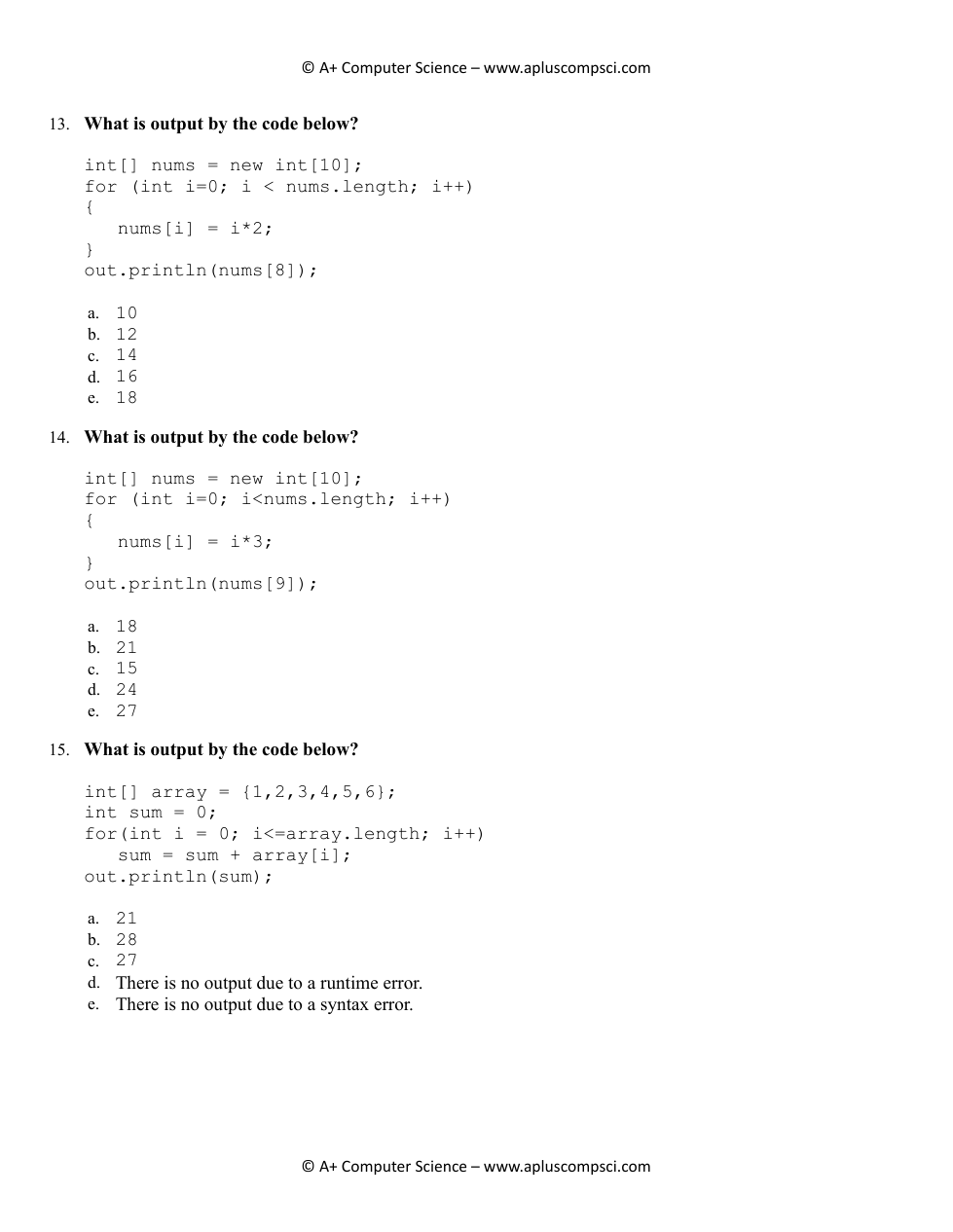
1 point
1
Question 13
13.
What is output by the code below?
int[] nums = new int[10];
for (int i=0; i < nums.length; i++) {
nums[i] = i*2;
}
out.println(nums[8]);
What is output by the code below?
int[] nums = new int[10];
for (int i=0; i < nums.length; i++)
{
nums[i] = i*2;
}
out.println(nums[8]);
1 point
1
Question 14
14.
What is output by the code below?
int[] nums = new int[10];
for (int i=0; i<nums.length; i++) {
nums[i] = i*3;
}
out.println(nums[9]);
What is output by the code below?
int[] nums = new int[10];
for (int i=0; i<nums.length; i++)
{
nums[i] = i*3;
}
out.println(nums[9]);
1 point
1
Question 15
15.
What is output by the code below?
int[] array = {1,2,3,4,5,6};
int sum = 0;
for(int i = 0; i<=array.length; i++)
sum = sum + array[i];
out.println(sum);
What is output by the code below?
int[] array = {1,2,3,4,5,6};
int sum = 0;
for(int i = 0; i<=array.length; i++)
sum = sum + array[i];
out.println(sum);
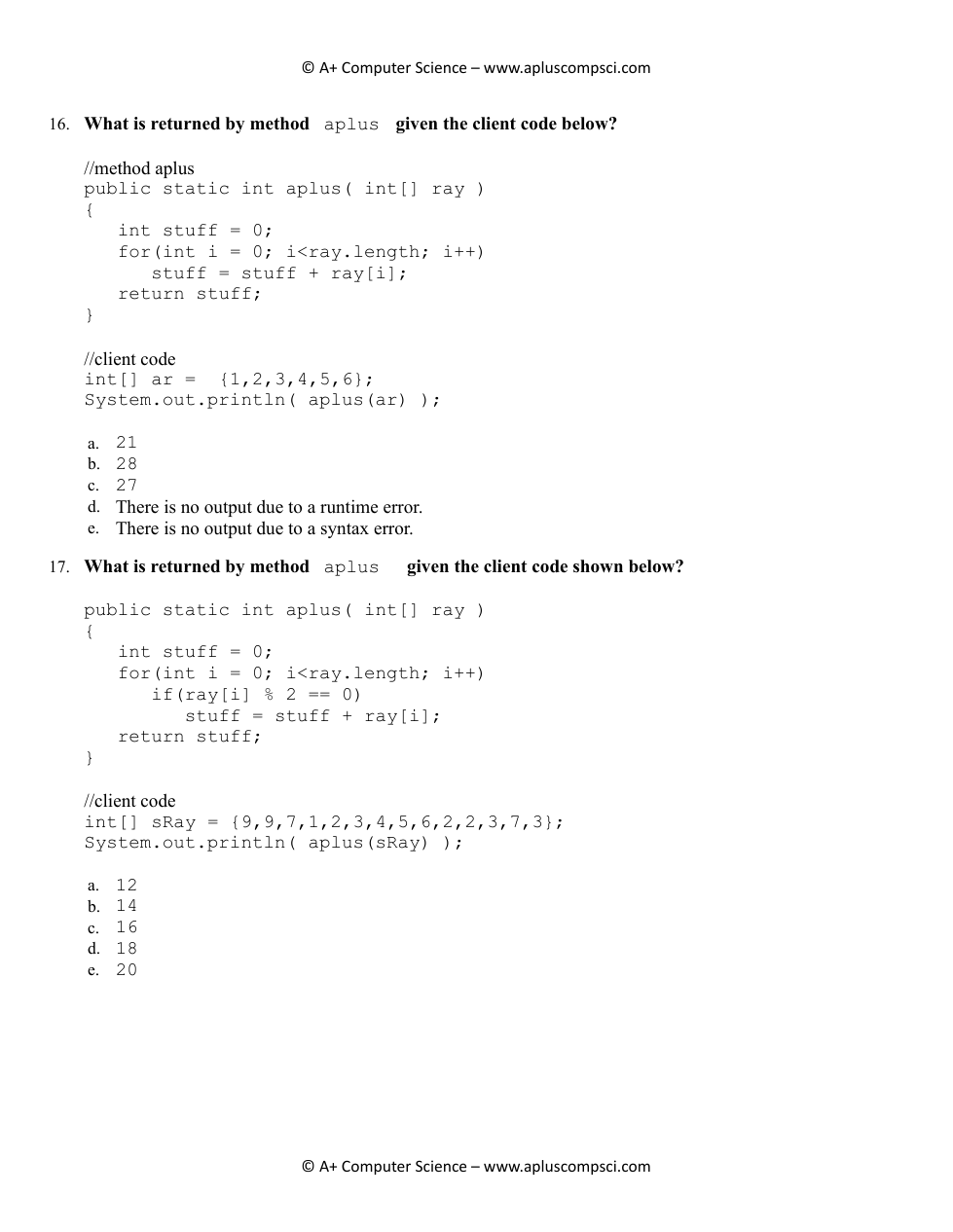
1 point
1
Question 16
16.
What is returned by method aplus given the client code below?
//method aplus
public static int aplus( int[] ray )
{
int stuff = 0;
for(int i = 0; i<ray.length; i++)
stuff = stuff + ray[i];
return stuff;
}
//client code
int[] ar = {1,2,3,4,5,6};
System.out.println( aplus(ar) );
What is returned by method aplus given the client code below?
//method aplus
public static int aplus( int[] ray )
{
int stuff = 0;
for(int i = 0; i<ray.length; i++)
stuff = stuff + ray[i];
return stuff;
}
//client code
int[] ar = {1,2,3,4,5,6};
System.out.println( aplus(ar) );
1 point
1
Question 17
17.
What is returned by method aplus given the client code shown below?
public static int aplus( int[] ray )
{
int stuff = 0;
for(int i = 0; i<ray.length; i++)
if(ray[i] % 2 == 0)
stuff = stuff + ray[i];
return stuff;
}
//client code
int[] sRay = {9,9,7,1,2,3,4,5,6,2,2,3,7,3};
System.out.println( aplus(sRay) );
What is returned by method aplus given the client code shown below?
public static int aplus( int[] ray )
{
int stuff = 0;
for(int i = 0; i<ray.length; i++)
if(ray[i] % 2 == 0)
stuff = stuff + ray[i];
return stuff;
}
//client code
int[] sRay = {9,9,7,1,2,3,4,5,6,2,2,3,7,3};
System.out.println( aplus(sRay) );
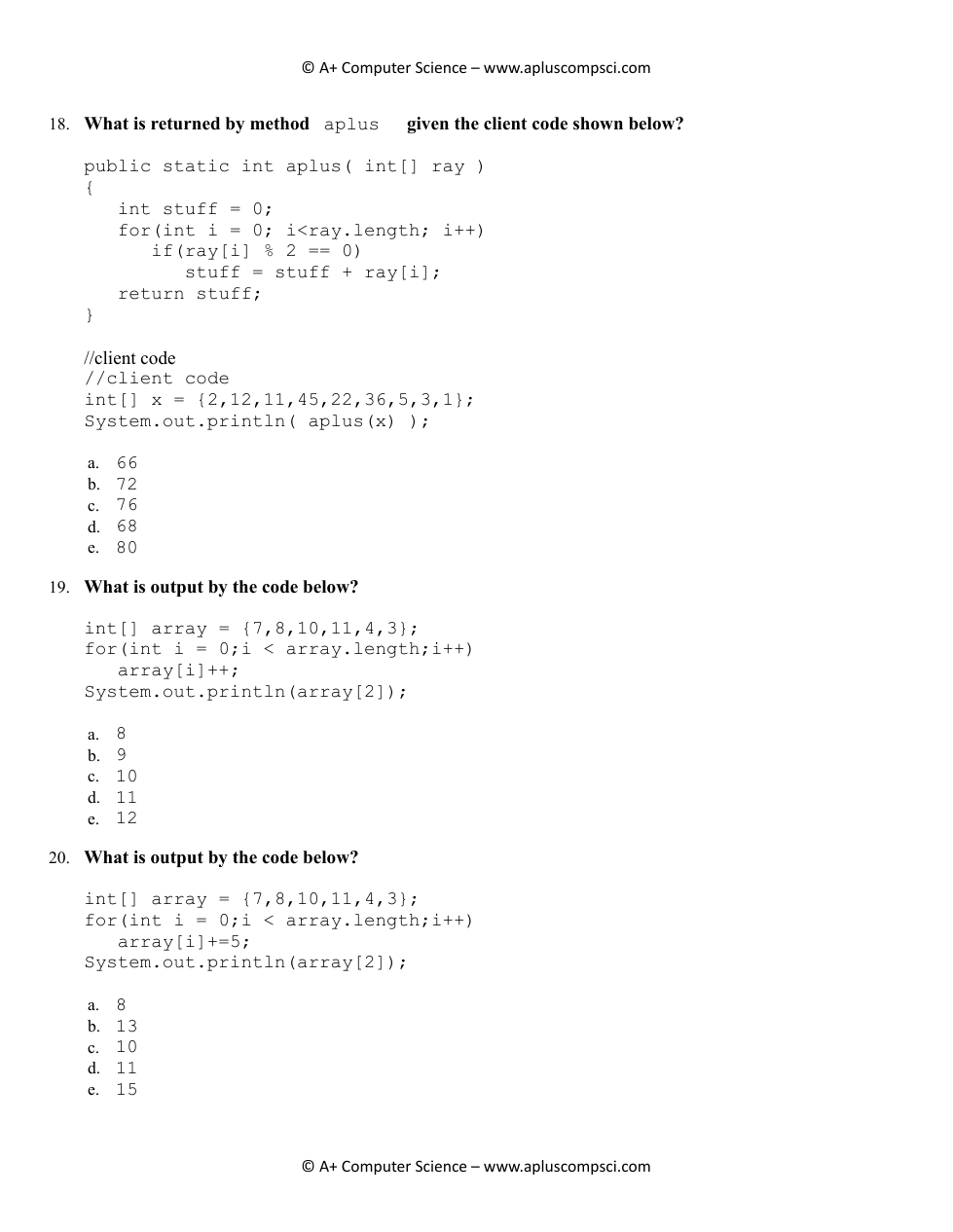
1 point
1
Question 18
18.
What is returned by method aplus given the client code shown below?
public static int aplus( int[] ray ) {
int stuff = 0;
for(int i = 0; i<ray.length; i++) {
if(ray[i] % 2 == 0) {
stuff = stuff + ray[i];
}
}
return stuff;
}
//client code
int[] x = {2,12,11,45,22,36,5,3,1};
System.out.println( aplus(x) );
What is returned by method aplus given the client code shown below?
public static int aplus( int[] ray ) {
int stuff = 0;
for(int i = 0; i<ray.length; i++) {
if(ray[i] % 2 == 0) {
stuff = stuff + ray[i];
}
}
return stuff;
}
//client code
int[] x = {2,12,11,45,22,36,5,3,1};
System.out.println( aplus(x) );
1 point
1
Question 19
19.
What is output by the code below?
int[] array = {7,8,10,11,4,3};
for(int i = 0; i < array.length; i++) {
array[i]++;
}
System.out.println(array[2]);
What is output by the code below?
int[] array = {7,8,10,11,4,3};
for(int i = 0; i < array.length; i++) {
array[i]++;
}
System.out.println(array[2]);
1 point
1
Question 20
20.
What is output by the code below?
int[] array = {7,8,10,11,4,3};
for(int i = 0; i < array.length; i++) {
array[i]+=5;
}
System.out.println(array[2]);
What is output by the code below?
int[] array = {7,8,10,11,4,3};
for(int i = 0; i < array.length; i++) {
array[i]+=5;
}
System.out.println(array[2]);
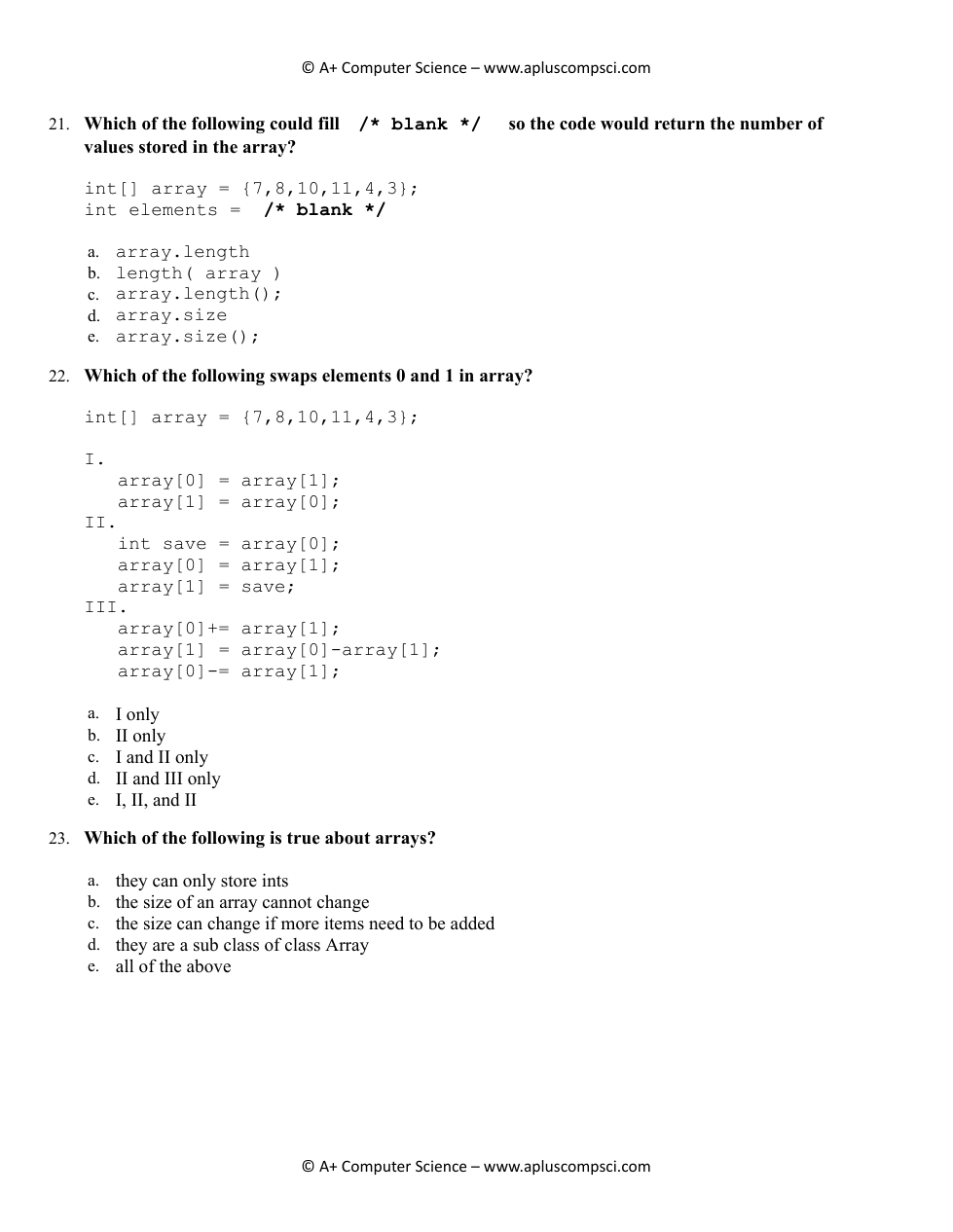
1 point
1
Question 21
21.
Which of the following could fill the blank so the code would return the number of values stored in the array?
int[] array = {7,8,10,11,4,3};
int elements = /* blank */
Which of the following could fill the blank so the code would return the number of values stored in the array?
int[] array = {7,8,10,11,4,3};
int elements = /* blank */
1 point
1
Question 22
22.
Which of the following swaps elements 0 and 1 in array?
int[] array = {7,8,10,11,4,3};
I.
array[0] = array[1];
array[1] = array[0];
II.
int save = array[0];
array[0] = array[1];
array[1] = save;
III.
array[0]+= array[1];
array[1] = array[0]-array[1];
array[0]-= array[1];
Which of the following swaps elements 0 and 1 in array?
int[] array = {7,8,10,11,4,3};
I.
array[0] = array[1];
array[1] = array[0];
II.
int save = array[0];
array[0] = array[1];
array[1] = save;
III.
array[0]+= array[1];
array[1] = array[0]-array[1];
array[0]-= array[1];
1 point
1
Question 23
23.
Which of the following is true about arrays?
Which of the following is true about arrays?
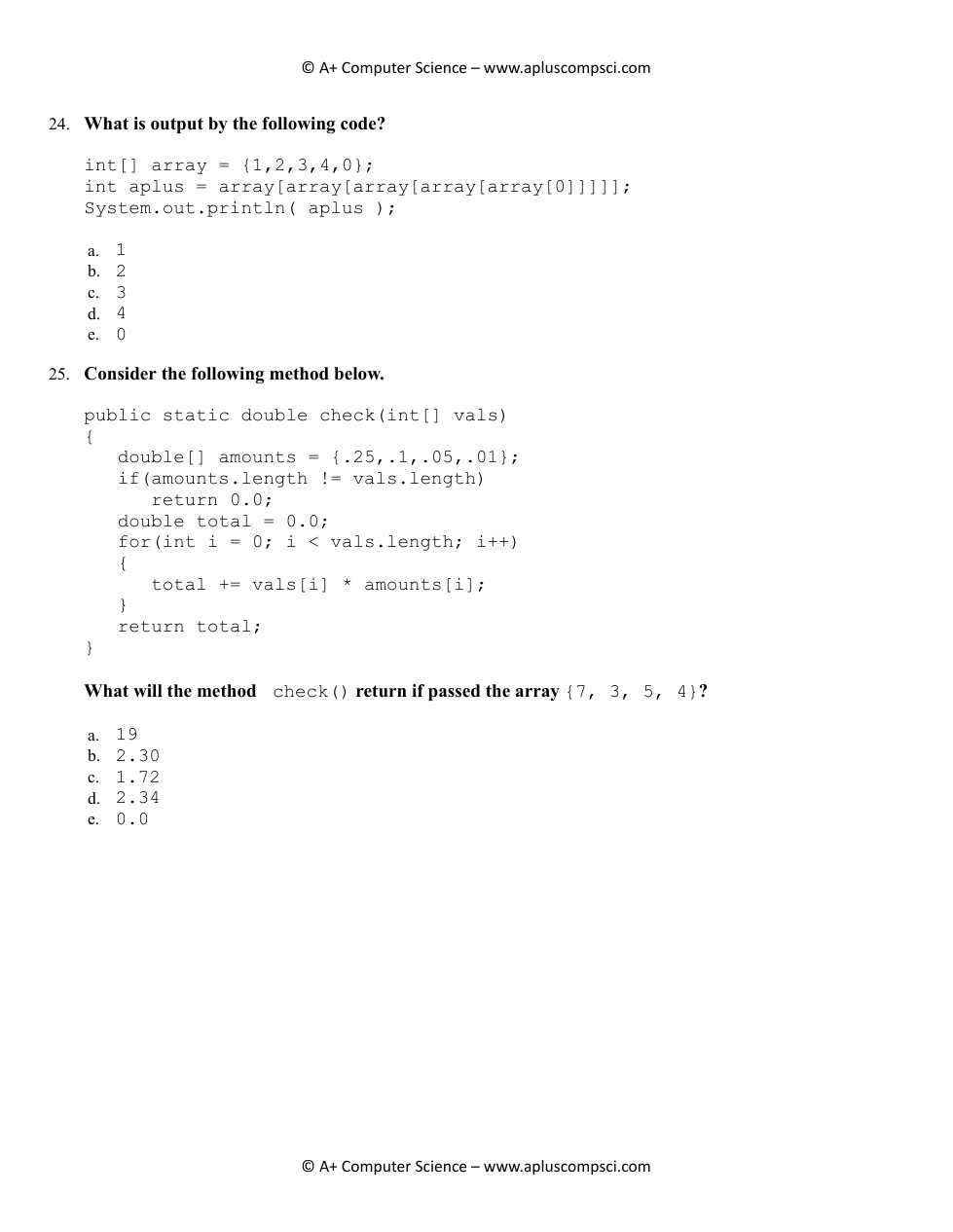
1 point
1
Question 24
24.
Question 24: What is output by the following code?
int[] array = {1,2,3,4,0};
int aplus = array[array[array[array[array[0]]]]];
System.out.println(aplus);
Question 24: What is output by the following code?
int[] array = {1,2,3,4,0};
int aplus = array[array[array[array[array[0]]]]];
System.out.println(aplus);
1 point
1
Question 25
25.
Question 25: Consider the following method below.
public static double check(int[] vals)
{
double[] amounts = {.25,.1,.05,.01};
if(amounts.length != vals.length)
return 0.0;
double total = 0.0;
for(int i = 0; i < vals.length; i++)
{
total += vals[i] * amounts[i];
}
return total;
}
What will the method check() return if passed the array {7, 3, 5, 4}?
Question 25: Consider the following method below.
public static double check(int[] vals)
{
double[] amounts = {.25,.1,.05,.01};
if(amounts.length != vals.length)
return 0.0;
double total = 0.0;
for(int i = 0; i < vals.length; i++)
{
total += vals[i] * amounts[i];
}
return total;
}
What will the method check() return if passed the array {7, 3, 5, 4}?
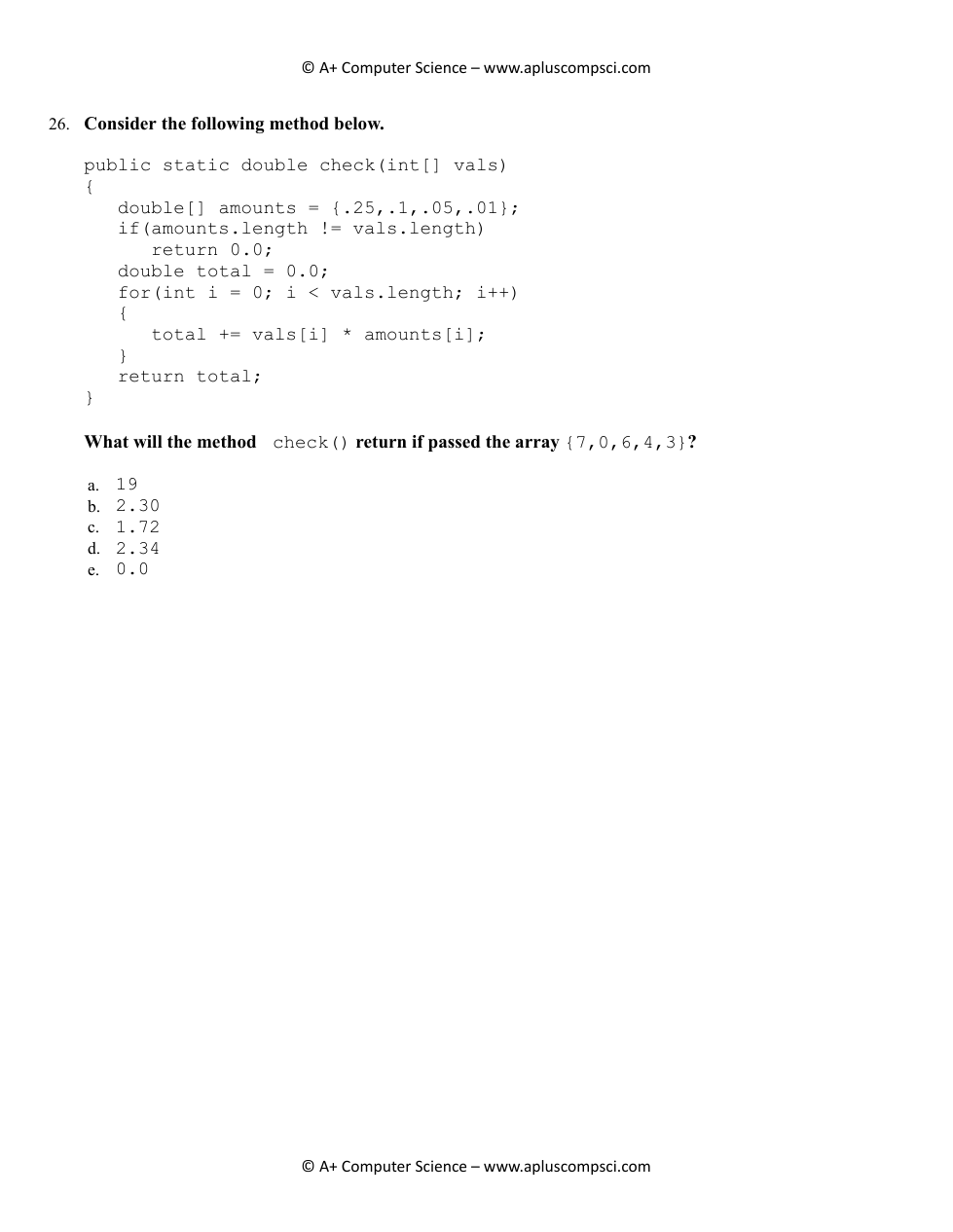
1 point
1
Question 26
26.
Consider the following method below.
public static double check(int[] vals)
{
double[] amounts = {.25,.1,.05,.01};
if(amounts.length != vals.length)
return 0.0;
double total = 0.0;
for(int i = 0; i < vals.length; i++)
{
total += vals[i] * amounts[i];
}
return total;
}
What will the method check() return if passed the array {7,0,6,4,3} ?
Consider the following method below.
public static double check(int[] vals)
{
double[] amounts = {.25,.1,.05,.01};
if(amounts.length != vals.length)
return 0.0;
double total = 0.0;
for(int i = 0; i < vals.length; i++)
{
total += vals[i] * amounts[i];
}
return total;
}
What will the method check() return if passed the array {7,0,6,4,3} ?
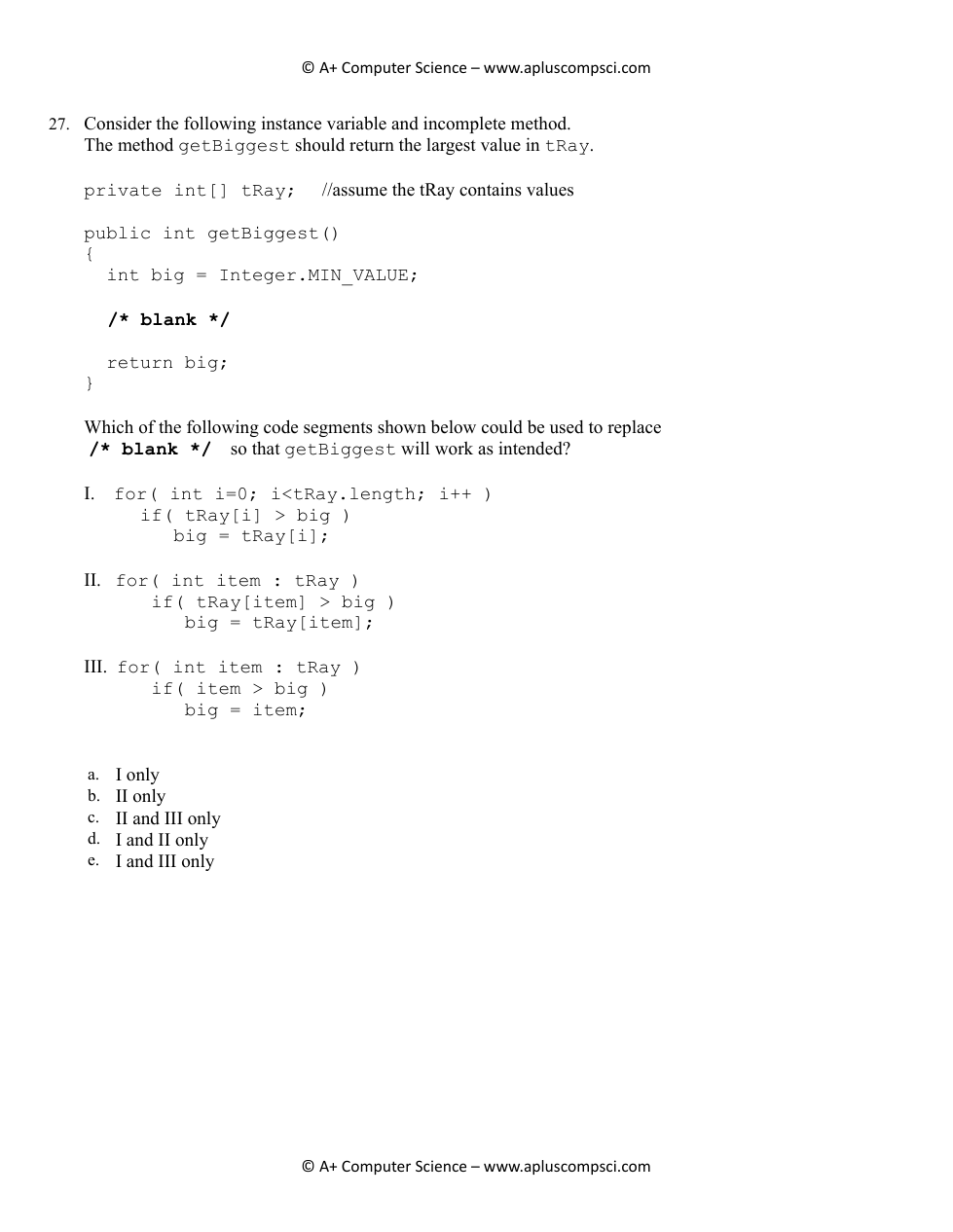
1 point
1
Question 27
27.
Consider the following instance variable and incomplete method. The method getBiggest should return the largest value in tRay.
private int[] tRay; // assume the tRay contains values
public int getBiggest() {
int big = Integer.MIN_VALUE;
/* blank */
return big;
}
Which of the following code segments shown below could be used to replace /* blank */ so that getBiggest will work as intended?I. for (int i = 0; i < tRay.length; i++)
if (tRay[i] > big) big = tRay[i];
II. for (int item : tRay) if (tRay[item] > big) big = tRay[item];
III. for (int item : tRay) if (item > big) big = item;
Consider the following instance variable and incomplete method. The method getBiggest should return the largest value in tRay.
private int[] tRay; // assume the tRay contains values
public int getBiggest() {
int big = Integer.MIN_VALUE;
/* blank */
return big;
}
Which of the following code segments shown below could be used to replace /* blank */ so that getBiggest will work as intended?
I. for (int i = 0; i < tRay.length; i++)
if (tRay[i] > big)
big = tRay[i];
II. for (int item : tRay)
if (tRay[item] > big)
big = tRay[item];
III. for (int item : tRay)
if (item > big)
big = item;
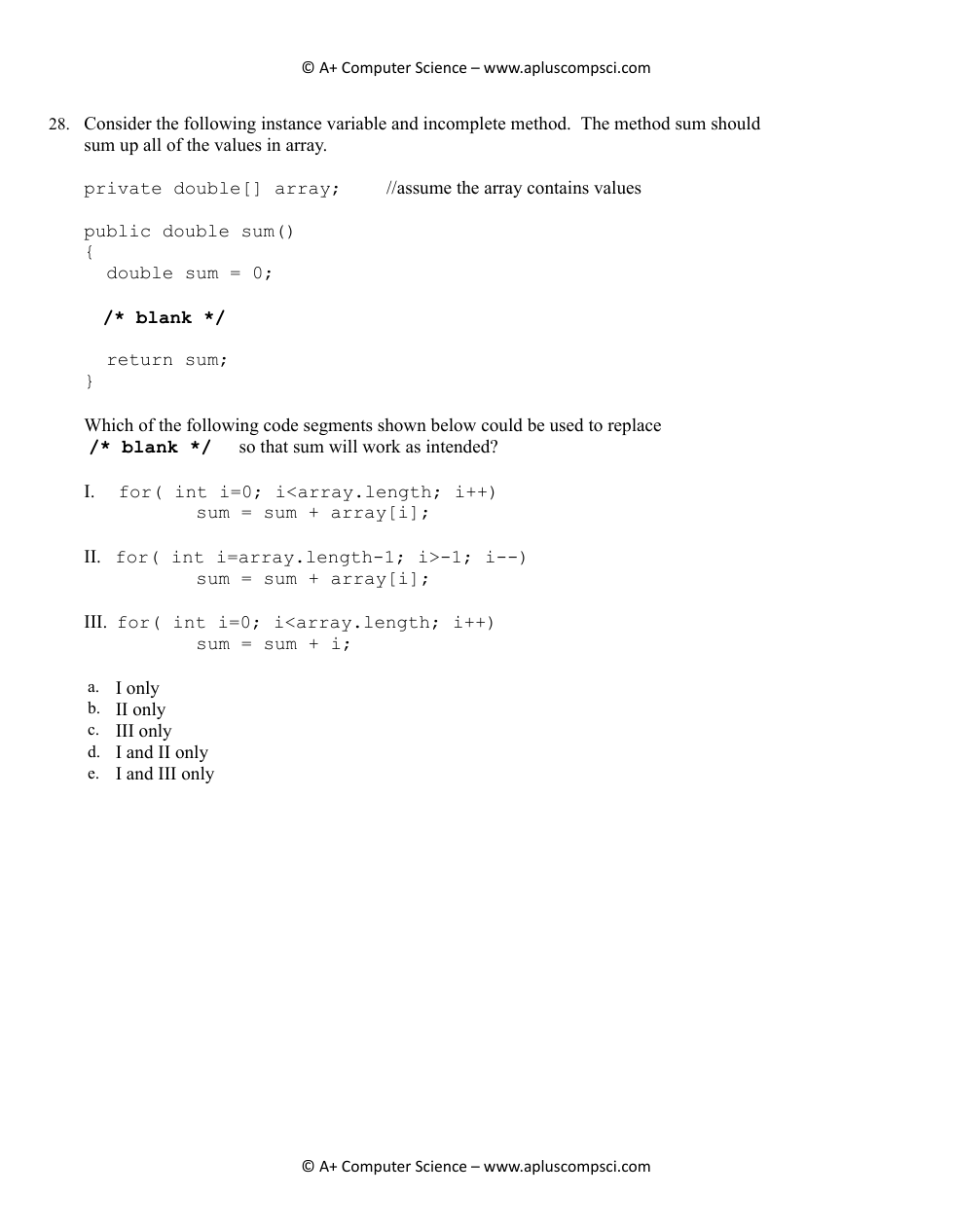
1 point
1
Question 28
28.
Consider the following instance variable and incomplete method. The method sum should sum up all of the values in array.
private double[] array; //assume the array contains values
public double sum()
{
double sum = 0;
/* blank */
return sum;
}
Which of the following code segments shown below could be used to replace /* blank */ so that sum will work as intended?
I. for( int i=0; i<array.length; i++) sum = sum + array[i];
II. for( int i=array.length-1; i>-1; i--) sum = sum + array[i];
III. for( int i=0; i<array.length; i++) sum = sum + i;
Consider the following instance variable and incomplete method. The method sum should sum up all of the values in array.
private double[] array; //assume the array contains values
public double sum()
{
double sum = 0;
/* blank */
return sum;
}
Which of the following code segments shown below could be used to replace /* blank */ so that sum will work as intended?
I. for( int i=0; i<array.length; i++)
sum = sum + array[i];
II. for( int i=array.length-1; i>-1; i--)
sum = sum + array[i];
III. for( int i=0; i<array.length; i++)
sum = sum + i;
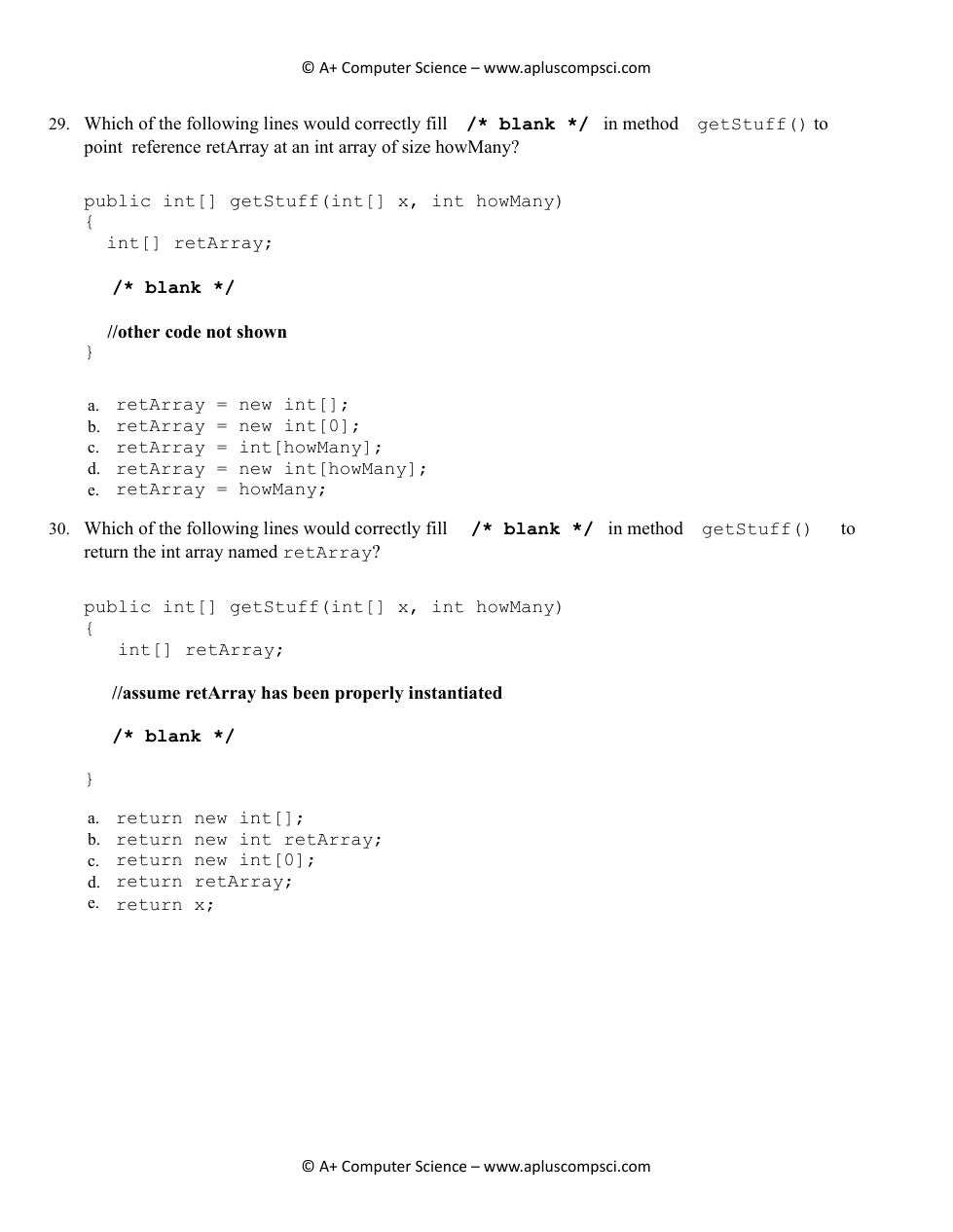
1 point
1
Question 29
29.
Which of the following lines would correctly fill the blank in method getStuff() to point reference retArray at an int array of size howMany?
public int [] get Stuff(int [] x, int howMany){
int [] retArray; /* blank *\
///other code not shown
}
Which of the following lines would correctly fill the blank in method getStuff() to point reference retArray at an int array of size howMany?
public int [] get Stuff(int [] x, int howMany){
int [] retArray;
/* blank *\
///other code not shown
}
1 point
1
Question 30
30.
Which of the following lines would correctly fill the blank in method getStuff() to return the int array named retArray?
public int [] getStuff(int [] x, int howMany){ int [] retArray; //assume retArray has been properly
instantiated
/*blank*/}
Which of the following lines would correctly fill the blank in method getStuff() to return the int array named retArray?
public int [] getStuff(int [] x, int howMany)
{
int [] retArray;
//assume retArray has been properly
instantiated
/*blank*/
}
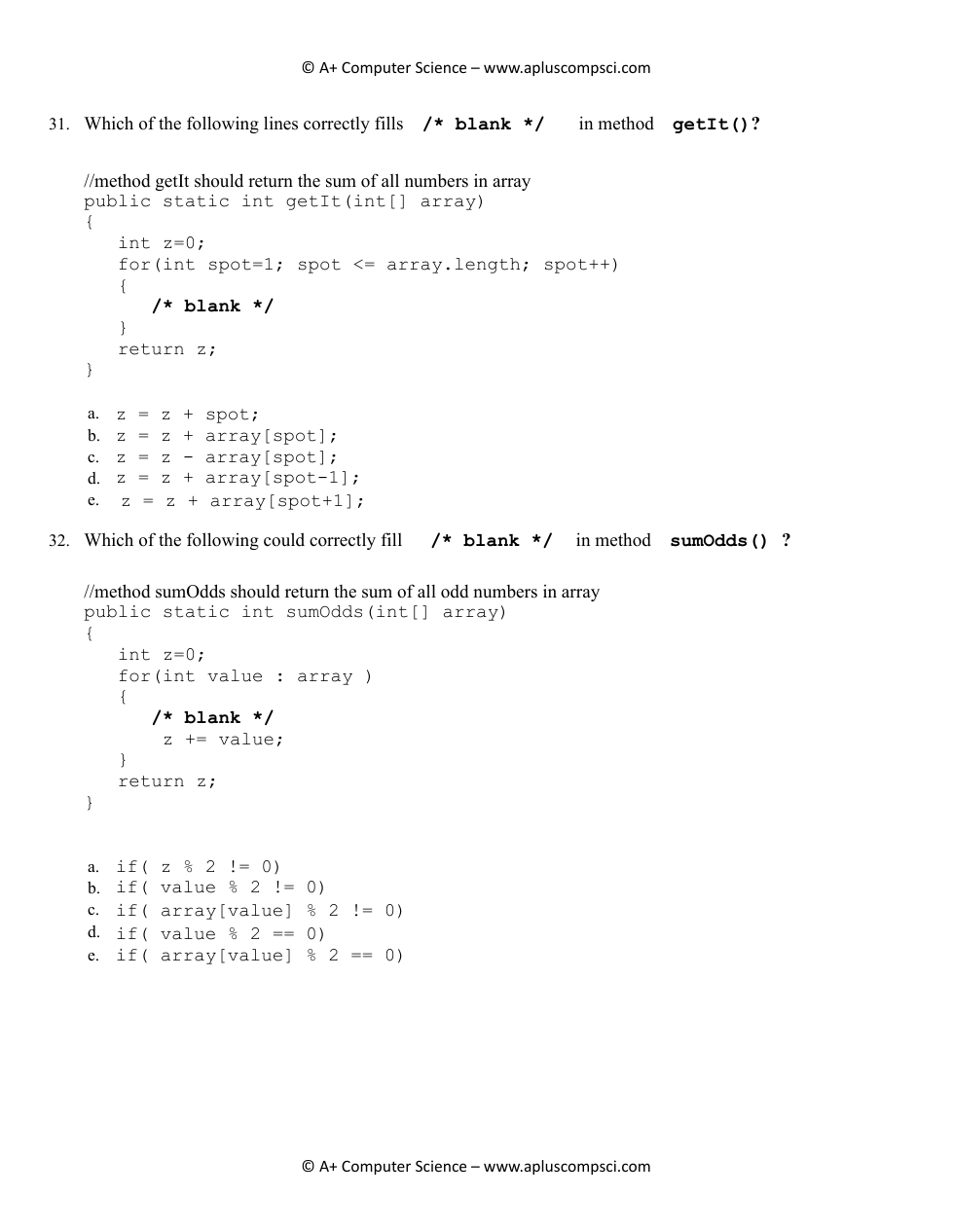
1 point
1
Question 31
31.
Question 31: Which of the following lines correctly fills in method getIt()?
//method getIt should return the sum of all numbers in array
public static int getIt(int[] array)
{
int z=0;
for(int spot=1; spot <= array.length; spot++)
{
/* blank */
}
return z;
}
Question 31: Which of the following lines correctly fills in method getIt()?
//method getIt should return the sum of all numbers in array
public static int getIt(int[] array)
{
int z=0;
for(int spot=1; spot <= array.length; spot++)
{
/* blank */
}
return z;
}
1 point
1
Question 32
32.
Question 32: Which of the following could correctly fill in method sumOdds()?
//method sumOdds should return the sum of all odd numbers in array
public static int sumOdds(int[] array)
{
int z=0;
for(int value : array )
{
/* blank */
z += value;
}
return z;
}
Question 32: Which of the following could correctly fill in method sumOdds()?
//method sumOdds should return the sum of all odd numbers in array
public static int sumOdds(int[] array)
{
int z=0;
for(int value : array )
{
/* blank */
z += value;
}
return z;
}
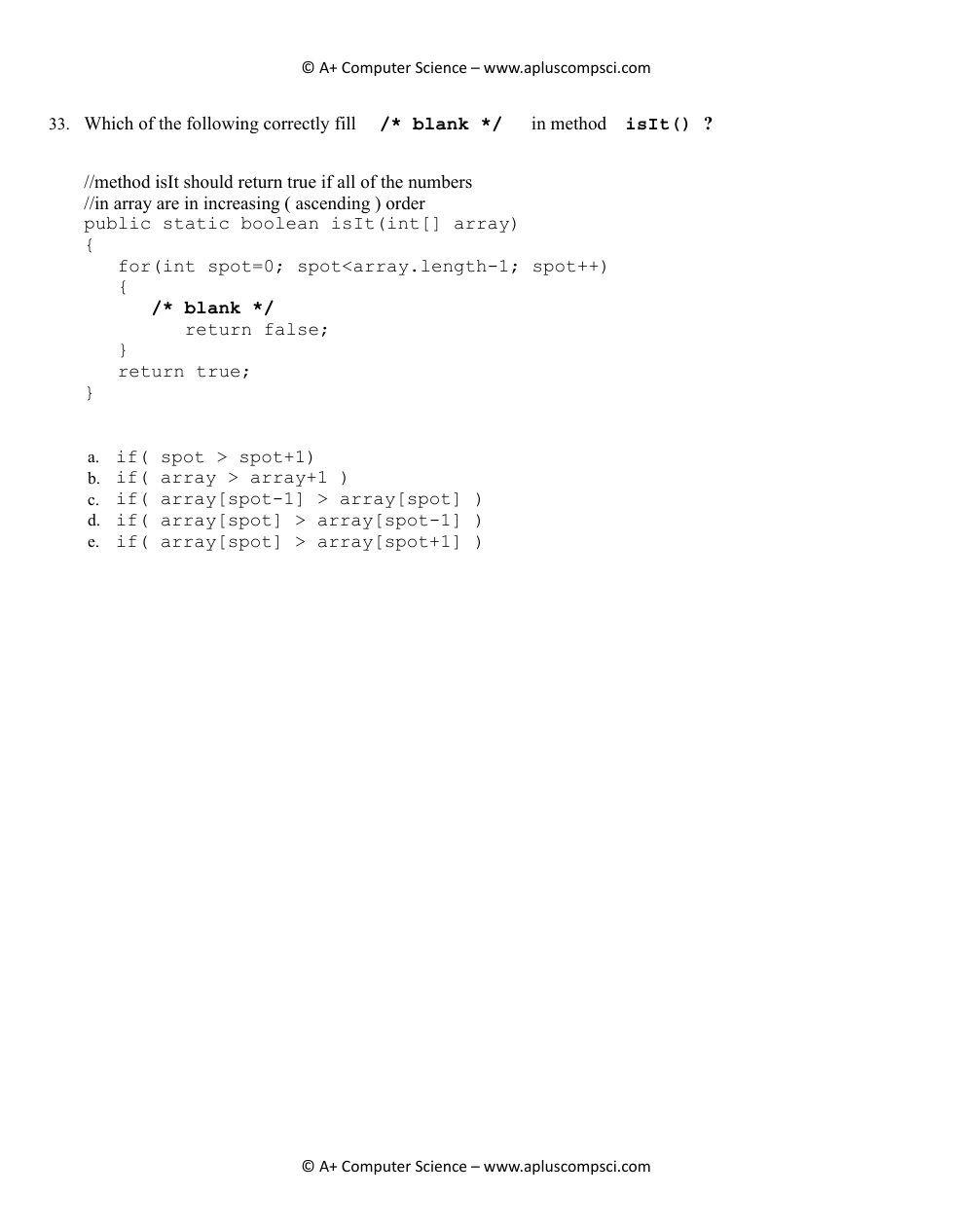
1 point
1
Question 33
33.
Which of the following correctly fill /* blank */ in method isIt() ?
//method isIt should return true if all of the numbers
//in array are in increasing (ascending) order
public static boolean isIt(int[] array) {
for(int spot=0; spot<array.length-1; spot++) {
/* blank */
return false;
}
return true;
}
Which of the following correctly fill /* blank */ in method isIt() ?
//method isIt should return true if all of the numbers
//in array are in increasing (ascending) order
public static boolean isIt(int[] array) {
for(int spot=0; spot<array.length-1; spot++)
{
/* blank */
return false;
}
return true;
}
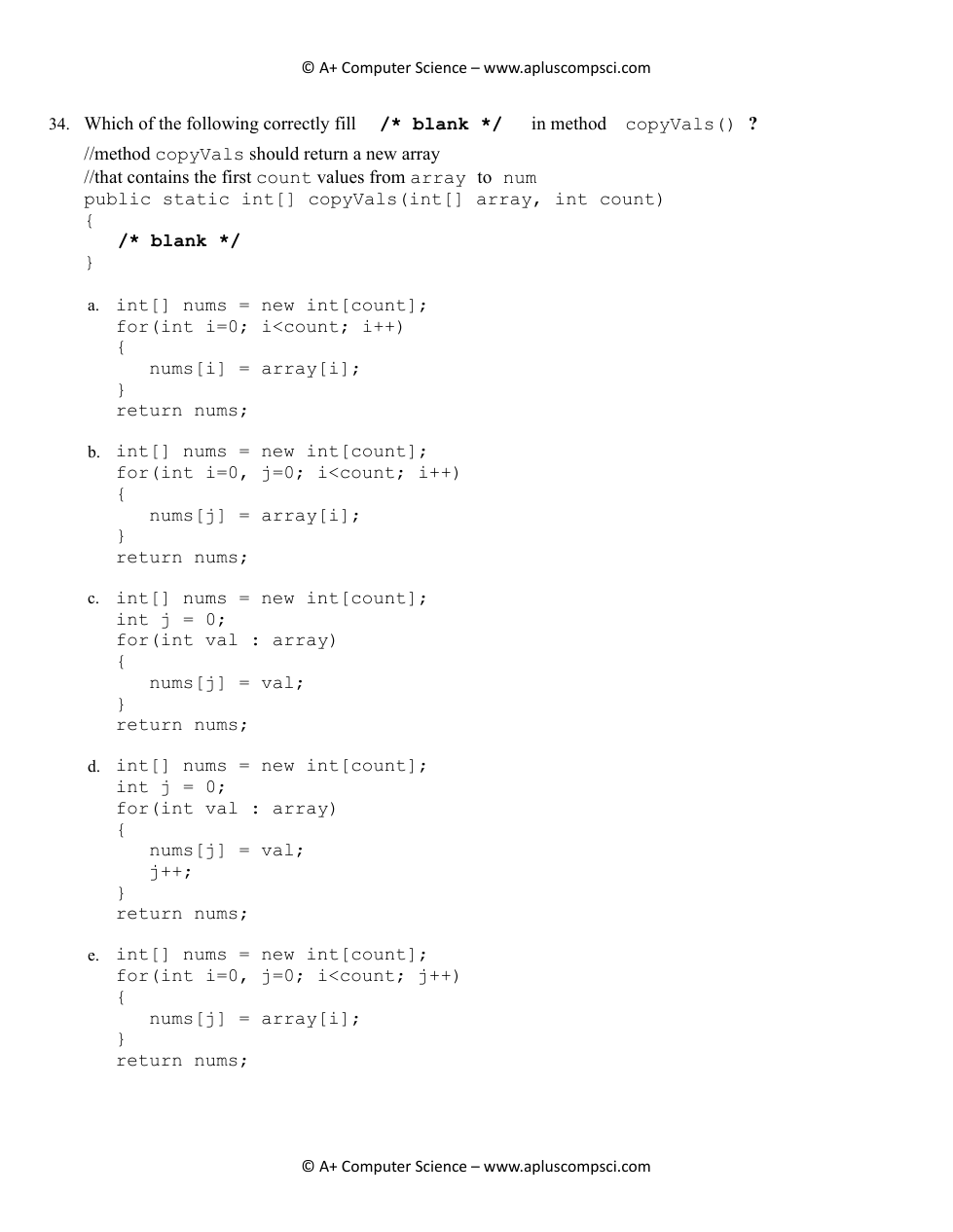
1 point
1
Question 34
34.
Which of the following correctly fill /* blank */ in method copyVals()?
//method copyVals should return a new array
//that contains the first count values from array to num
public static int[] copyVals(int[] array, int count)
{
/* blank */
}
Which of the following correctly fill /* blank */ in method copyVals()?
//method copyVals should return a new array
//that contains the first count values from array to num
public static int[] copyVals(int[] array, int count)
{
/* blank */
}
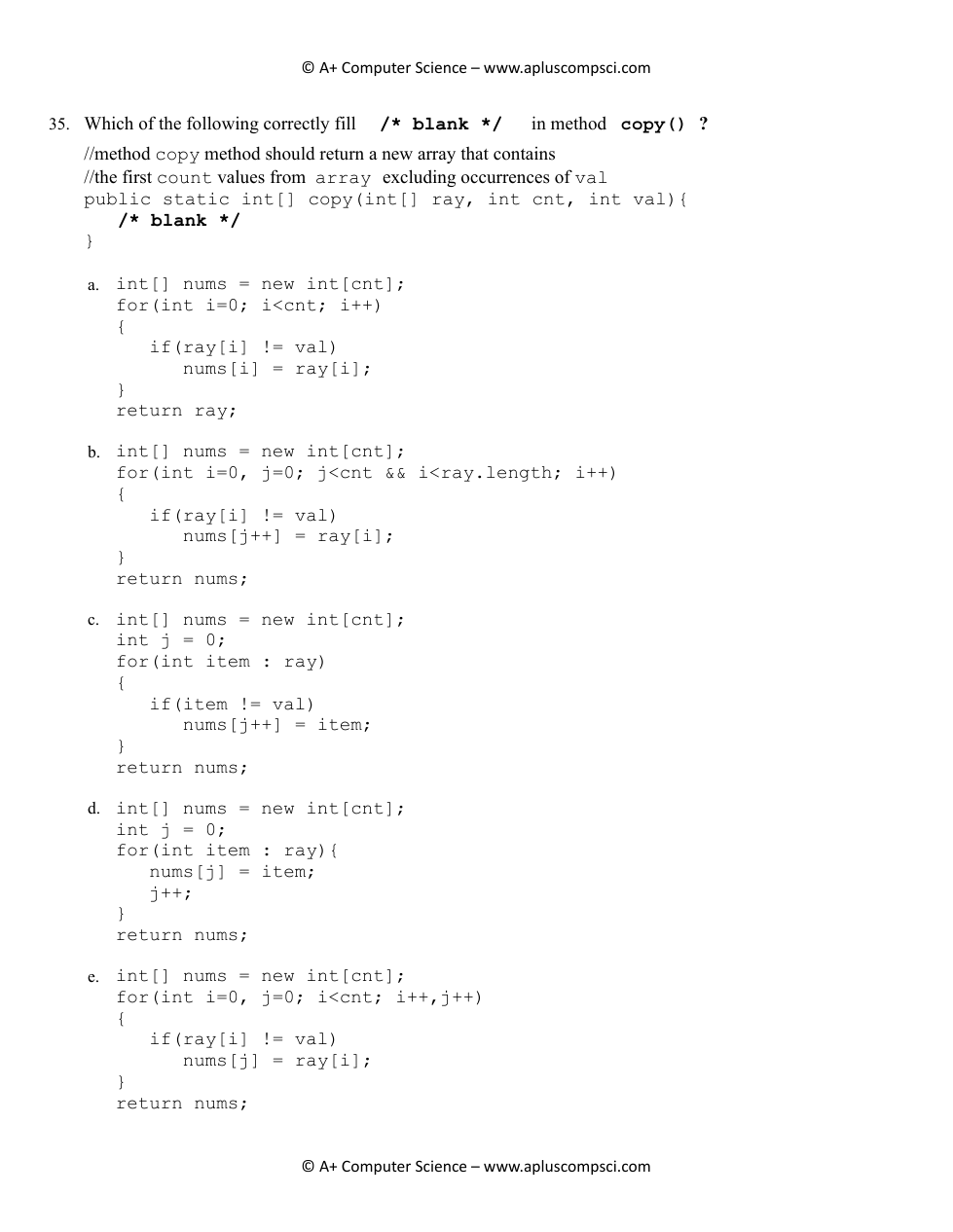
1 point
1
Question 35
35.
Question 35: Which of the following correctly fill in method copy()?
//method copy method should return a new array that contains
//the first count values from array excluding occurrences of val
public static int[] copy(int[] ray, int cnt, int val){
/* blank */
}
a. int[] nums = new int[cnt];
for(int i=0; i<cnt; i++)
{
if(ray[i] != val)
nums[i] = ray[i];
}
return ray;
b. int[] nums = new int[cnt];
for(int i=0, j=0; j<cnt && i<ray.length; i++)
{
if(ray[i] != val)
nums[j++] = ray[i];
}
return nums;
c. int[] nums = new int[cnt];
int j = 0;
for(int item : ray)
{
if(item != val)
nums[j++] = item;
}
return nums;
d. int[] nums = new int[cnt];
int j = 0;
for(int item : ray){
nums[j] = item;
j++;
}
return nums;
e. int[] nums = new int[cnt];
for(int i=0, j=0; i<cnt; i++,j++)
{
if(ray[i] != val)
nums[j] = ray[i];
}
return nums;
Question 35: Which of the following correctly fill in method copy()?
//method copy method should return a new array that contains
//the first count values from array excluding occurrences of val
public static int[] copy(int[] ray, int cnt, int val){
/* blank */
}
a. int[] nums = new int[cnt];
for(int i=0; i<cnt; i++)
{
if(ray[i] != val)
nums[i] = ray[i];
}
return ray;
b. int[] nums = new int[cnt];
for(int i=0, j=0; j<cnt && i<ray.length; i++)
{
if(ray[i] != val)
nums[j++] = ray[i];
}
return nums;
c. int[] nums = new int[cnt];
int j = 0;
for(int item : ray)
{
if(item != val)
nums[j++] = item;
}
return nums;
d. int[] nums = new int[cnt];
int j = 0;
for(int item : ray){
nums[j] = item;
j++;
}
return nums;
e. int[] nums = new int[cnt];
for(int i=0, j=0; i<cnt; i++,j++)
{
if(ray[i] != val)
nums[j] = ray[i];
}
return nums;
1 point
1
Question 36
36.
Consider the following instance variable and incomplete method. The method go shouldreturn the difference between the smallest and largest values in the array. The array willcontain values that range from Integer.MIN_VALUE to Integer.MAX_VALUE.private int[] array; //assume the array contains valuespublic int go(){int diff = 0;/* blank */return diff;}Which of the following code segments shown below could be used to replace/* blank */ so that method go will work as intended?I.int small = 0, big = 0;for( int i=0; i<array.length; i++){ if( small < array[i] ) small = array[i]; if( big > array[i] ) big = array[i];}diff = big - small;II.int small = Integer.MAX_VALUE;int big = Integer.MIN_VALUE;for( int i=0; i<array.length; i++){ if( small > array[i] ) small = array[i]; if( big < array[i] ) big = array[i];}diff = big - small;III.int small = Integer.MAX_VALUE;int big = Integer.MIN_VALUE;for( int i=0; i<array.length; i++){ if( small < array[i] ) small = array[i]; if( big > array[i] ) big = array[i];}diff = big - small;
Consider the following instance variable and incomplete method. The method go should
return the difference between the smallest and largest values in the array. The array will
contain values that range from Integer.MIN_VALUE to Integer.MAX_VALUE.
private int[] array; //assume the array contains values
public int go()
{
int diff = 0;
/* blank */
return diff;
}
Which of the following code segments shown below could be used to replace
/* blank */ so that method go will work as intended?
I.
int small = 0, big = 0;
for( int i=0; i<array.length; i++)
{
if( small < array[i] )
small = array[i];
if( big > array[i] )
big = array[i];
}
diff = big - small;
II.
int small = Integer.MAX_VALUE;
int big = Integer.MIN_VALUE;
for( int i=0; i<array.length; i++)
{
if( small > array[i] )
small = array[i];
if( big < array[i] )
big = array[i];
}
diff = big - small;
III.
int small = Integer.MAX_VALUE;
int big = Integer.MIN_VALUE;
for( int i=0; i<array.length; i++)
{
if( small < array[i] )
small = array[i];
if( big > array[i] )
big = array[i];
}
diff = big - small;
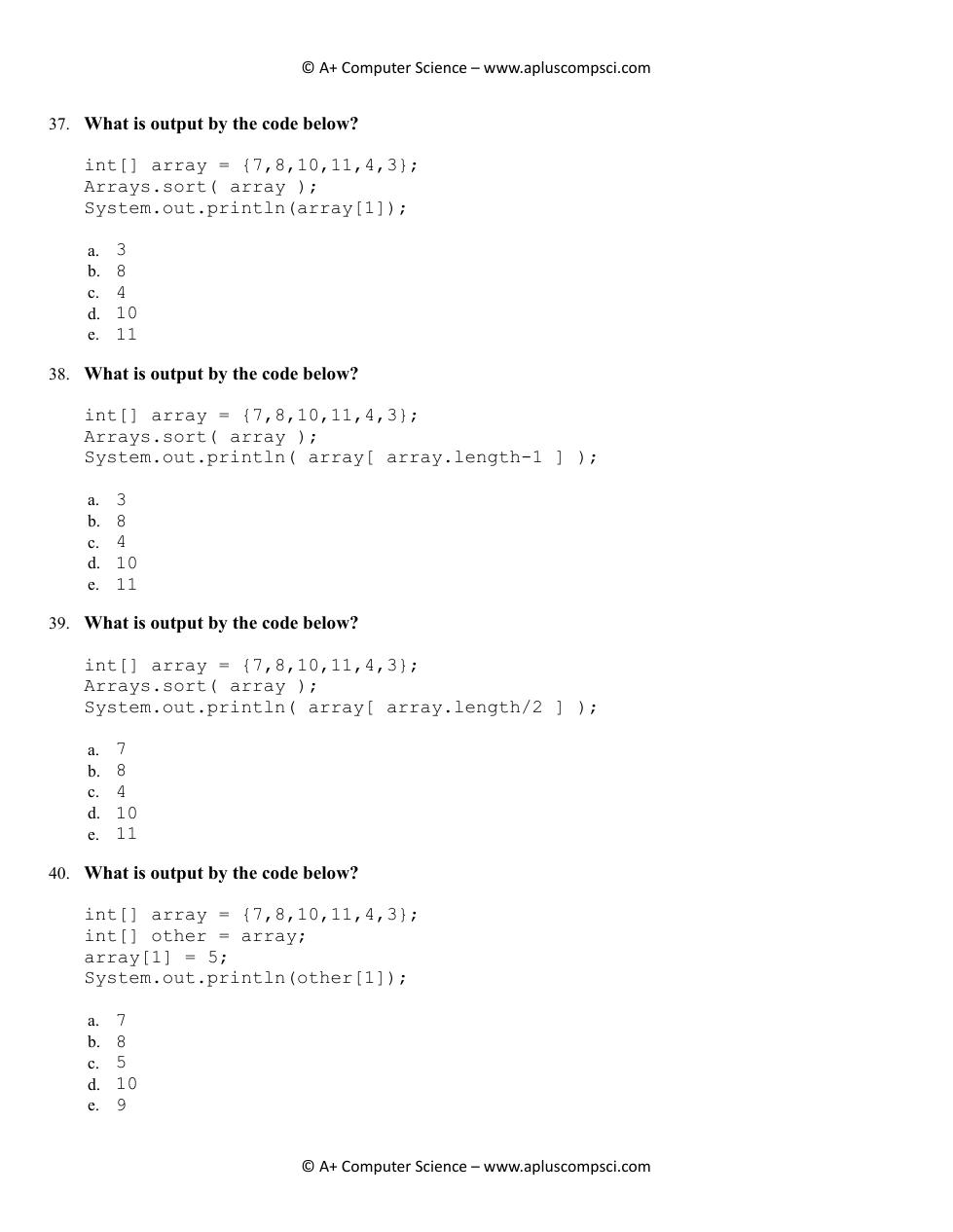
1 point
1
Question 37
37.
What is output by the code below? int[] array = {7,8,10,11,4,3}; Arrays.sort(array); System.out.println(array[1]);
What is output by the code below? int[] array = {7,8,10,11,4,3}; Arrays.sort(array); System.out.println(array[1]);
1 point
1
Question 38
38.
What is output by the code below? int[] array = {7,8,10,11,4,3}; Arrays.sort(array); System.out.println(array[array.length-1]);
What is output by the code below? int[] array = {7,8,10,11,4,3}; Arrays.sort(array); System.out.println(array[array.length-1]);
1 point
1
Question 39
39.
What is output by the code below? int[] array = {7,8,10,11,4,3}; Arrays.sort(array); System.out.println(array[array.length/2]);
What is output by the code below? int[] array = {7,8,10,11,4,3}; Arrays.sort(array); System.out.println(array[array.length/2]);
1 point
1
Question 40
40.
What is output by the code below? int[] array = {7,8,10,11,4,3}; int[] other = array; array[1] = 5; System.out.println(other[1]);
What is output by the code below? int[] array = {7,8,10,11,4,3}; int[] other = array; array[1] = 5; System.out.println(other[1]);