Array of Ref Test
By Mickey Arnold
starstarstarstarstarstarstarstarstarstar
Last updated 10 months ago
12 Questions
5 points
5
Question 1
1.
What is output by the code below?
String[] ray;ray = new String[4];System.out.println( ray[0] );
What is output by the code below?
String[] ray;
ray = new String[4];
System.out.println( ray[0] );
5 points
5
Question 2
2.
What is output by the code below?
Integer[] ray;ray = new Integer[4];System.out.println( ray[3] );
What is output by the code below?
Integer[] ray;
ray = new Integer[4];
System.out.println( ray[3] );
5 points
5
Question 3
3.
What is output by the code below?
Integer[] ray;ray = new Integer[4];ray[1] = null;ray[0] = new Integer(3);ray[2] = new Integer(99);System.out.println( ray[2] );
What is output by the code below?
Integer[] ray;
ray = new Integer[4];
ray[1] = null;
ray[0] = new Integer(3);
ray[2] = new Integer(99);
System.out.println( ray[2] );
5 points
5
Question 4
4.
What is output by the code below?
Integer[] ray = {11,55,-4,909};System.out.println( ray[3] );
What is output by the code below?
Integer[] ray = {11,55,-4,909};
System.out.println( ray[3] );
5 points
5
Question 5
5.
What is output by the code below?
Double[] ray = {11.2,55.0,-4.5,909.0};System.out.println( ray[1] );
What is output by the code below?
Double[] ray = {11.2,55.0,-4.5,909.0};
System.out.println( ray[1] );
5 points
5
Question 6
6.
What is output by the code below?
Double[] ray = {11.2,7.2,-4.5,909.0};ray[1] = new Double(55.0);System.out.println( ray[1] );
What is output by the code below?
Double[] ray = {11.2,7.2,-4.5,909.0};
ray[1] = new Double(55.0);
System.out.println( ray[1] );
5 points
5
Question 7
7.
What is output by the code below?
Double[] ray = {3.2,5.7,6.3,1.9};double x = 0;for( double r : ray ) x += r;System.out.println( x );
What is output by the code below?
Double[] ray = {3.2,5.7,6.3,1.9};
double x = 0;
for( double r : ray )
x += r;
System.out.println( x );
5 points
5
Question 8
8.
What is output by the code below?
Double[] ray = {3.1,5.2,6.3,1.4};for( double r : ray ) r = 0;System.out.println( ray[2] );
What is output by the code below?
Double[] ray = {3.1,5.2,6.3,1.4};
for( double r : ray )
r = 0;
System.out.println( ray[2] );
5 points
5
Question 9
9.
What is output by the code below?
String[] ray;ray = new String[4];ray[1] = "one";System.out.println( ray[1] );
What is output by the code below?
String[] ray;
ray = new String[4];
ray[1] = "one";
System.out.println( ray[1] );
5 points
5
Question 10
10.
What is output by the code below?
String[] ray;ray = new String[4];ray[1] = "one";ray[2] = "two";ray[3] = "three";System.out.println( ray[0] );
What is output by the code below?
String[] ray;
ray = new String[4];
ray[1] = "one";
ray[2] = "two";
ray[3] = "three";
System.out.println( ray[0] );
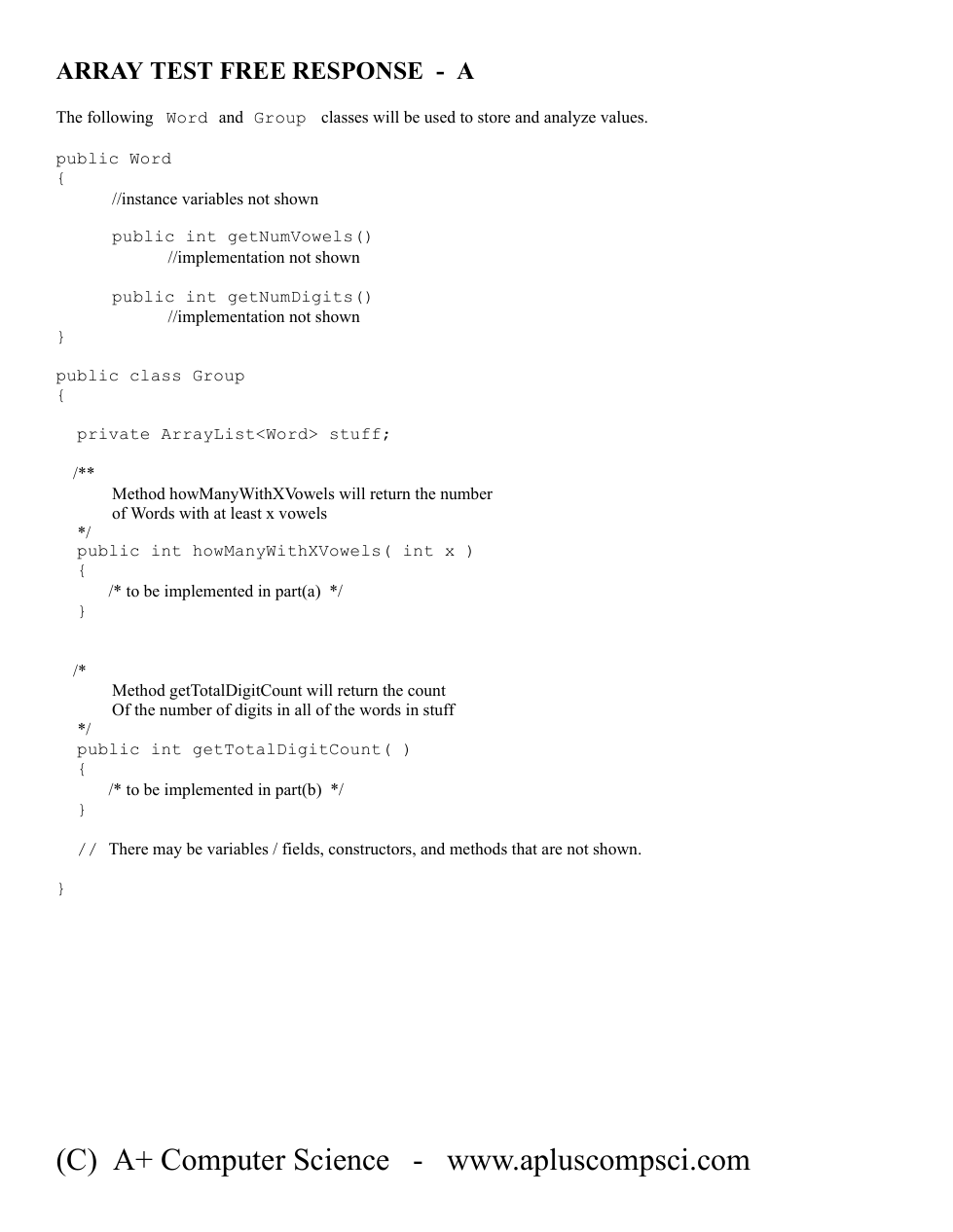
25 points
25
Question 11
11.
Part A. Write the Group method howManyWithXVowels(), as started below. howManyWithXVowels will receive an integer and determine if each word has more than that number of vowels.
If the list is ["about","dogs","123456","A"]
The call howManyWithXVowels( 1 ) would return 3
The call howManyWithXVowels( 3 ) would return 1
/**Method howManyWithXVowels will return the numberof Words with at least x vowels*/
public int howManyWithXVowels( int x ){
//Code goes here//
}
Part A. Write the Group method howManyWithXVowels(), as started below. howManyWithXVowels will receive an integer and determine if each word has more than that number of vowels.
If the list is ["about","dogs","123456","A"]
The call howManyWithXVowels( 1 ) would return 3
The call howManyWithXVowels( 3 ) would return 1
/**
Method howManyWithXVowels will return the number
of Words with at least x vowels
*/
public int howManyWithXVowels( int x )
{
//Code goes here//
}
25 points
25
Question 12
12.
Part B. Write the Group method getTotalDigitCount( ), as started below. getTotalDigitCount( ) will return the total number of digits in all of the words in the list.
If the list is ["abou7","dogs","123456","A","34Funny"]
The call getTotalDigitCount( ) would return 9
/*Method getTotalDigitCount will return the countOf the number of digits in all of the words in stuff*/
public int getTotalDigitCount( ){
//Code goes here//
}
Part B. Write the Group method getTotalDigitCount( ), as started below. getTotalDigitCount( ) will return the total number of digits in all of the words in the list.
If the list is ["abou7","dogs","123456","A","34Funny"]
The call getTotalDigitCount( ) would return 9
/*
Method getTotalDigitCount will return the count
Of the number of digits in all of the words in stuff
*/
public int getTotalDigitCount( )
{
//Code goes here//
}