Arraylist Test
By Mickey Arnold
starstarstarstarstarstarstarstarstarstar
Last updated 10 months ago
13 Questions
1 point
1
Question 1
1.
Which of the following code segments could fill /* blank */ so the code would add the first and third element of list?
ArrayList<Object> list = new ArrayList<Object>();
list.add(5);
list.add("abc");
list.add(4);
list.add(1.2);
list.add('a');
list.add("10");
int i = /* blank */
Which of the following code segments could fill /* blank */ so the code would add the first and third element of list?
ArrayList<Object> list = new ArrayList<Object>();
list.add(5);
list.add("abc");
list.add(4);
list.add(1.2);
list.add('a');
list.add("10");
int i = /* blank */
1 point
1
Question 2
2.
What is output by the code below?
String[] ray;
ray = new String[4];
ray[1] = "one";
ray[2] = "two";
ray[3] = "three";
ray[0] = ray[2];
ray[2] = null;
System.out.println( ray[0] );
What is output by the code below?
String[] ray;
ray = new String[4];
ray[1] = "one";
ray[2] = "two";
ray[3] = "three";
ray[0] = ray[2];
ray[2] = null;
System.out.println( ray[0] );
1 point
1
Question 3
3.
Which of the descriptions provided below describes the best way to improve the code shown below to ensure that all of the occurrences of string one will be removed?public static void removeAllOnes(ArrayList<String> all){ int spot = all.size() - 1; while ( spot > 0 ) { if( all.get(spot).equals("one") ) all.remove(spot); spot--; }}
Which of the descriptions provided below describes the best way to improve the code shown below to ensure that all of the occurrences of string one will be removed?
public static void removeAllOnes(ArrayList<String> all)
{
int spot = all.size() - 1;
while ( spot > 0 )
{
if( all.get(spot).equals("one") )
all.remove(spot);
spot--;
}
}
1 point
1
Question 4
4.
What is output by the code below?
List<String> bigList = new ArrayList<String>();
bigList.add(0,"one");
bigList.add("two");
bigList.add(0,"three");
bigList.add("four");
bigList.add(0,"five");
bigList.set(2,"six");
bigList.set(3,"seven");
out.println( bigList.indexOf("three") );
What is output by the code below?
List<String> bigList = new ArrayList<String>();
bigList.add(0,"one");
bigList.add("two");
bigList.add(0,"three");
bigList.add("four");
bigList.add(0,"five");
bigList.set(2,"six");
bigList.set(3,"seven");
out.println( bigList.indexOf("three") );
1 point
1
Question 5
5.
Consider the following class definitions.public class Cat{ private String name; private int age;
public String getName() //implementation not shown public int getAge() //implementation not shown //constructors and other methods not shown}
public class CatBarn{ private Cat[] kitties; //assume kitties has stuff in it
//returns the number of kittes older than > age public int countCats( int age ){ /* blank */ }//constructors and other methods not shown}
Which of the following code segments shown below could be used to replace/* blank */ so that method countCats() will work as intended?I.int cnt = 0;for( Cat c : kitties ) if( c > age ) cnt = cnt + 1;return cnt;
II.int cnt = 0;for( Cat c : kitties ) if( c.getAge() > age ) cnt = cnt + 1;return cnt;
III.int cnt = 0;for( int i = 0; i < kitties.length; i++ ) if( kitties[i].getAge() > age ) cnt += 1;return cnt;
Consider the following class definitions.
public class Cat
{
private String name;
private int age;
public String getName()
//implementation not shown
public int getAge()
//implementation not shown
//constructors and other methods not shown
}
public class CatBarn
{
private Cat[] kitties; //assume kitties has stuff in it
//returns the number of kittes older than > age
public int countCats( int age ){
/* blank */
}
//constructors and other methods not shown
}
Which of the following code segments shown below could be used to replace
/* blank */ so that method countCats() will work as intended?
I.
int cnt = 0;
for( Cat c : kitties )
if( c > age )
cnt = cnt + 1;
return cnt;
II.
int cnt = 0;
for( Cat c : kitties )
if( c.getAge() > age )
cnt = cnt + 1;
return cnt;
III.
int cnt = 0;
for( int i = 0; i < kitties.length; i++ )
if( kitties[i].getAge() > age )
cnt += 1;
return cnt;
1 point
1
Question 6
6.
Which of the following code segments could fill /* blank */ so the code would retrieve the numeric value of the last element?
ArrayList<Object> list = new ArrayList<Object>();list.add(5);list.add("abc");list.add(4);list.add(1.2);list.add('a');list.add("10");
int i = /* blank */ ;
Which of the following code segments could fill /* blank */ so the code would retrieve the numeric value of the last element?
ArrayList<Object> list = new ArrayList<Object>();
list.add(5);
list.add("abc");
list.add(4);
list.add(1.2);
list.add('a');
list.add("10");
int i = /* blank */ ;
1 point
1
Question 7
7.
Method removeAllOnes should go through the entire ArrayList and remove all occurrences of the word one. Examine the code below and determine if the code works or if the code has issues. Select the best description of the code from the answer choices shown below.
public static void removeAllOnes(ArrayList<String> all){ for(int spot=0; spot < all.size(); spot++) { if( all.get(spot).equals("one") ) all.remove(spot); }}
Method removeAllOnes should go through the entire ArrayList and remove all occurrences of the word one. Examine the code below and determine if the code works or if the code has issues. Select the best description of the code from the answer choices shown below.
public static void removeAllOnes(ArrayList<String> all)
{
for(int spot=0; spot < all.size(); spot++)
{
if( all.get(spot).equals("one") )
all.remove(spot);
}
}
1 point
1
Question 8
8.
Consider the following Pig class and client code below.class Pig{ public Pig( int x, String y ) { //code not shown }}/////////////////////////////////////////////////////////////////////////////////////////////////////////////client codeArrayList< Pig > list;list = new ArrayList< Pig >();/* blank */
Which of the following could fill /* blank */ to add a Pig to the list?
I. list.add( 5 );II. list.add( 5, "piggy" );III. list.add( new Pig( 5, "piggy" ) ) ;
Consider the following Pig class and client code below.
class Pig
{
public Pig( int x, String y )
{
//code not shown
}
}
///////////////////////////////////////////////////////////////////////////////////////////////////////////
//client code
ArrayList< Pig > list;
list = new ArrayList< Pig >();
/* blank */
Which of the following could fill /* blank */ to add a Pig to the list?
I. list.add( 5 );
II. list.add( 5, "piggy" );
III. list.add( new Pig( 5, "piggy" ) ) ;
1 point
1
Question 9
9.
What is output by the code below?String[] ray;ray = new String[4];System.out.println( ray[0] );
What is output by the code below?
String[] ray;
ray = new String[4];
System.out.println( ray[0] );
1 point
1
Question 10
10.
Which of the following code segments could fill /* blank */ so the code would add the first and fourth element of list?
ArrayList<Object> list = new ArrayList<Object>();list.add(5);list.add("abc");list.add(4);list.add(1.2);list.add('a');list.add("10");
double i = /* blank */
Which of the following code segments could fill /* blank */ so the code would add the first and fourth element of list?
ArrayList<Object> list = new ArrayList<Object>();
list.add(5);
list.add("abc");
list.add(4);
list.add(1.2);
list.add('a');
list.add("10");
double i = /* blank */
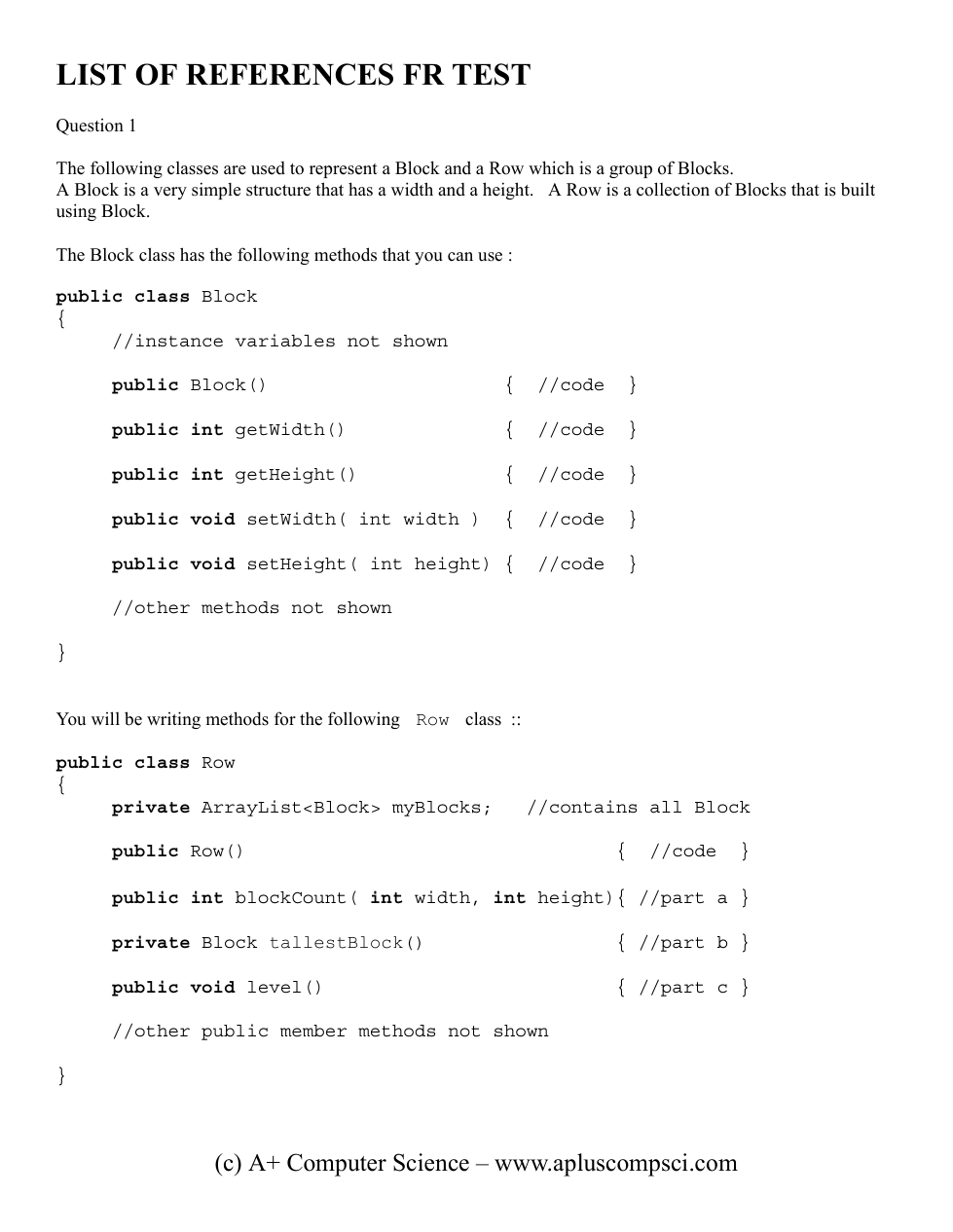
1 point
1
Question 11
11.
Part A. Write the Row method blockCount(), as started below. blockCount() will count up how many Blocks there are in the Row that have a width and height equal to the supplied parameters.
In writing blockCount() you may call any of the public methods of class Block and any of the public or private methods of class Row. Assume that all of the listed methods work as specified.
// This method counts up the number of Blocks with a height and width that match the provided params
public int blockCount( int width, int height ) {
//Code goes here//
}
Part A. Write the Row method blockCount(), as started below. blockCount() will count up how many Blocks there are in the Row that have a width and height equal to the supplied parameters.
In writing blockCount() you may call any of the public methods of class Block and any of the public or private methods of class Row. Assume that all of the listed methods work as specified.
// This method counts up the number of Blocks with a height and width that match the provided params
public int blockCount( int width, int height ) {
//Code goes here//
}
1 point
1
Question 12
12.
B. Write the Row method tallestBlock(), as started below. tallestBlock() will go through the entire ArrayList of Block and return a reference to the Block that is the tallest. The tallest Block would be the block with the greatest height.
In writing tallestBlock() you may call any of the public methods of the Block and Row classes. Assume that all the listed methods work as specified.
// This method will return a reference to the Block with the greatest height// myBlocks contains at least one Block
public Block tallestBlock(){
//Code goes here//
}
B. Write the Row method tallestBlock(), as started below. tallestBlock() will go through the entire ArrayList of Block and return a reference to the Block that is the tallest. The tallest Block would be the block with the greatest height.
In writing tallestBlock() you may call any of the public methods of the Block and Row classes. Assume that all the listed methods work as specified.
// This method will return a reference to the Block with the greatest height
// myBlocks contains at least one Block
public Block tallestBlock(){
//Code goes here//
}
1 point
1
Question 13
13.
Part C. Write the Row method level(), as started below. level() will go through the entire ArrayList of Block and make sure that the entire row has the same height as the tallest Block in the Row.
In writing level() you may call any of the public methods of class Block and any of the public or private methods of class Row. Assume that all the listed methods work as specified.
// This method would set all Block to the same height as the tallest Block
public void level() {
//Code goes here//
}
Part C. Write the Row method level(), as started below. level() will go through the entire ArrayList of Block and make sure that the entire row has the same height as the tallest Block in the Row.
In writing level() you may call any of the public methods of class Block and any of the public or private methods of class Row. Assume that all the listed methods work as specified.
// This method would set all Block to the same height as the tallest Block
public void level() {
//Code goes here//
}